Python .NET¶
Python can be used to interact with the PMP system. The easiest way to interface with the PMP API using Python is to use the Python.NET package and interface with the .NET PMP API. Python.NET is available for Windows and is compatible and tested with Python 3.7-3.12 (see http://pythonnet.github.io).
During this guide you will learn how to:
Setup your Python environment.
Interface with PMP API using Python .NET.
Requirements¶
The PMP C# API requires a .NET implementation that supports .NET Standard 2.0.
To check which version of .NET is installed, run the following in a command window:
wmic product where "Name like 'Microsoft .Net%'" get Name,Version
Note
This operation may take a few minutes.
To check your Python version, use the following in the windows command console:
python --version
Note
Make sure to add Python to the Windows PATH variable to execute python from a command window.
The PMP API is only available in 64-bit and a 64-bit version of Python is required to interface with the API. To verify you are using a 64-bit version of Python, use the following command:
python -c "import platform; print(platform.architecture()[0])"
To install Python.NET run the following in a command window:
pip install pythonnet
Project configuration¶
During this quick start guide, Visual Studio Code is used.
Open Visual Studio and select New File…
Select Python File.
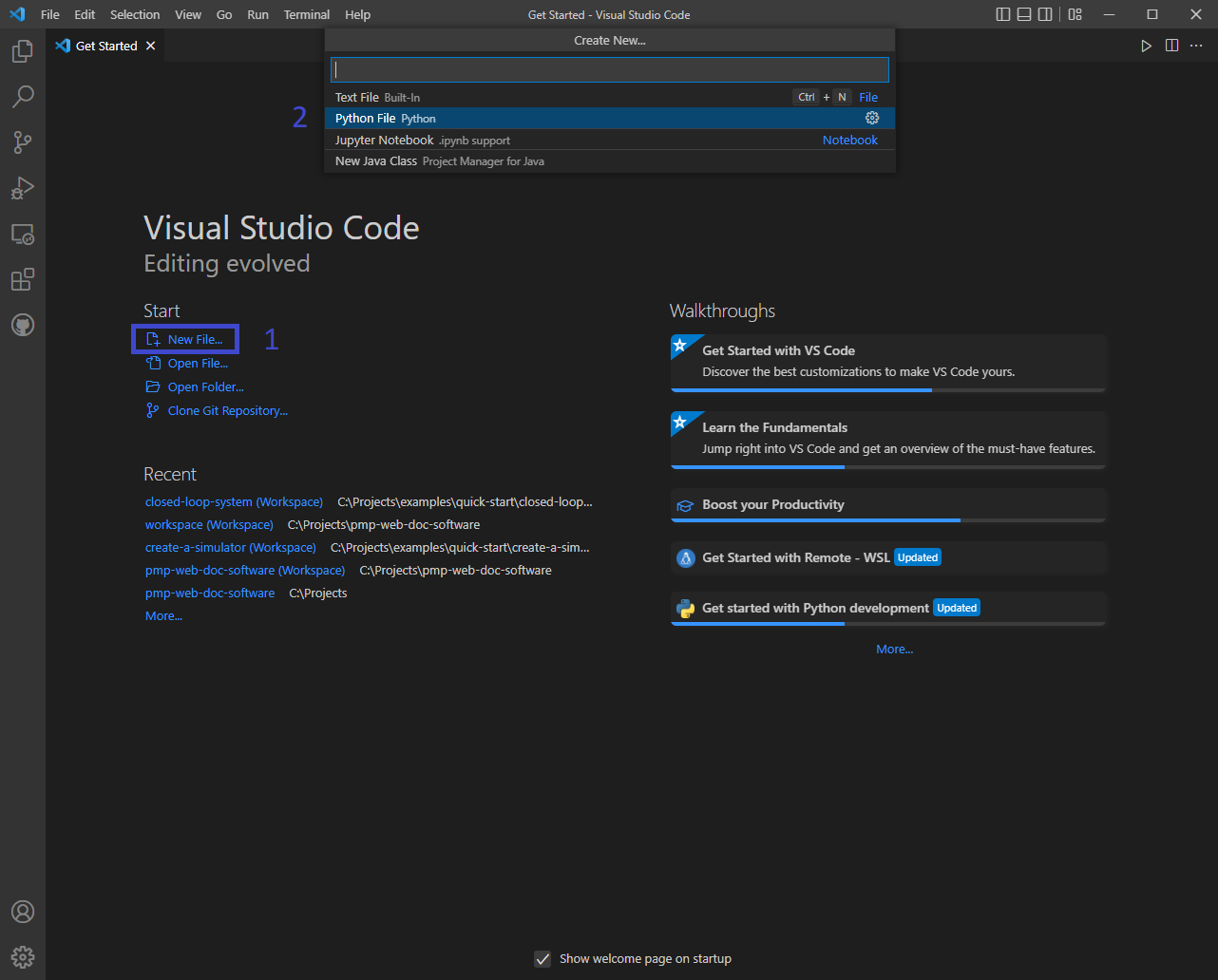
Start Python project in VS Code¶
Add .NET assembly¶
It is sufficient to add the pmpclient.net.dll to Python. The pmpclient.dll will automatically be called when placed in the same folder. The .NET assembly can be added via the following commands:
1 2 3 4 5 | import clr
clr.AddReference(
"C:\\Program Files\\Prodrive Motion Platform\\%PMP_VERSION%\\bin\\pmpclient.net.dll"
)
|
Example¶
Now that the project is fully configured, a PMP system instance can be created. To verify the system is functioning we can for example get the API version of the system as shown in the following code:
1 2 3 4 5 6 7 8 9 10 11 | import clr
clr.AddReference(
"C:\\Program Files\\Prodrive Motion Platform\\%PMP_VERSION%\\bin\\pmpclient.net.dll"
)
import Pmp
system = Pmp.System("localhost")
api_version = system.Version
print(f"PMP API version: {api_version.Major}.{api_version.Minor}.{api_version.Patch}")
|
First, make sure to select the same Python interpreter version in VS Code as defined in Windows PATH, since VS Code uses by default the newest Python version. To select a Python interpreter, type Ctrl-Shift-P, click on Python: Select Interpreter, and select the correct Python version.
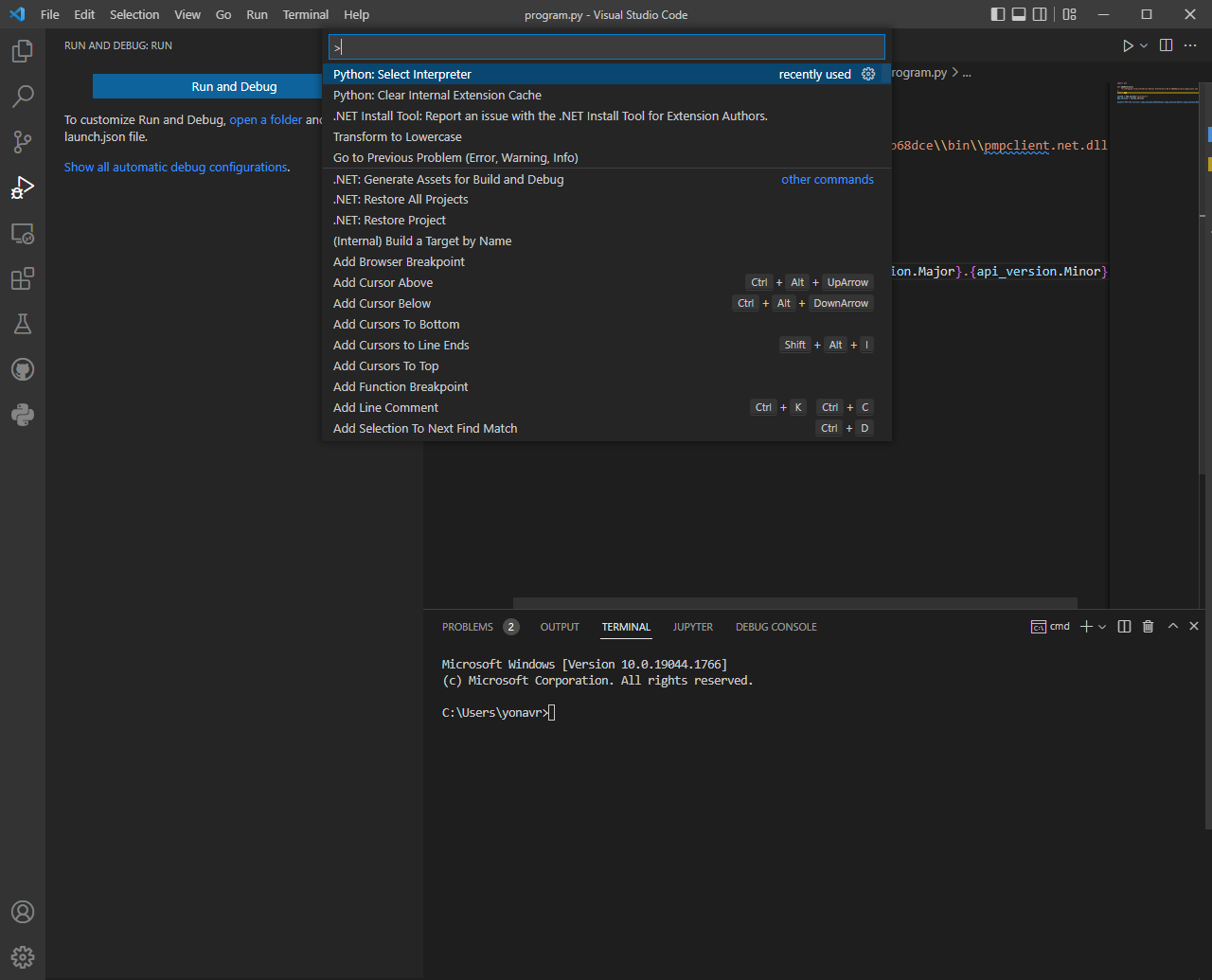
Select Python interpreter¶
To run the program:
From the sidebar select Run and Debug and press on Run and Debug (Or use F5 to initialize debugging).
In the debug configuration list, select Python File
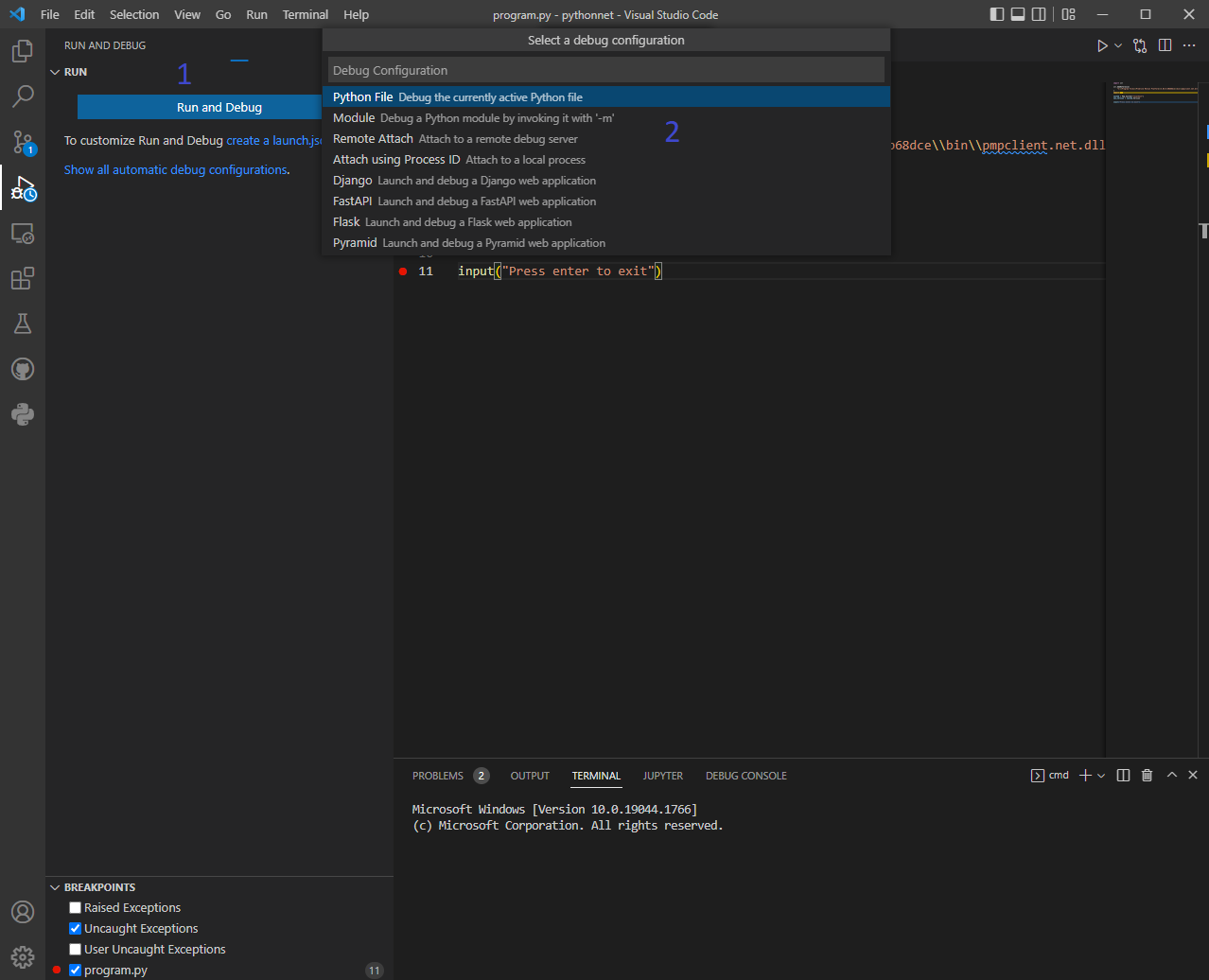
Run python program in debug¶
The API version is displayed in the terminal output:
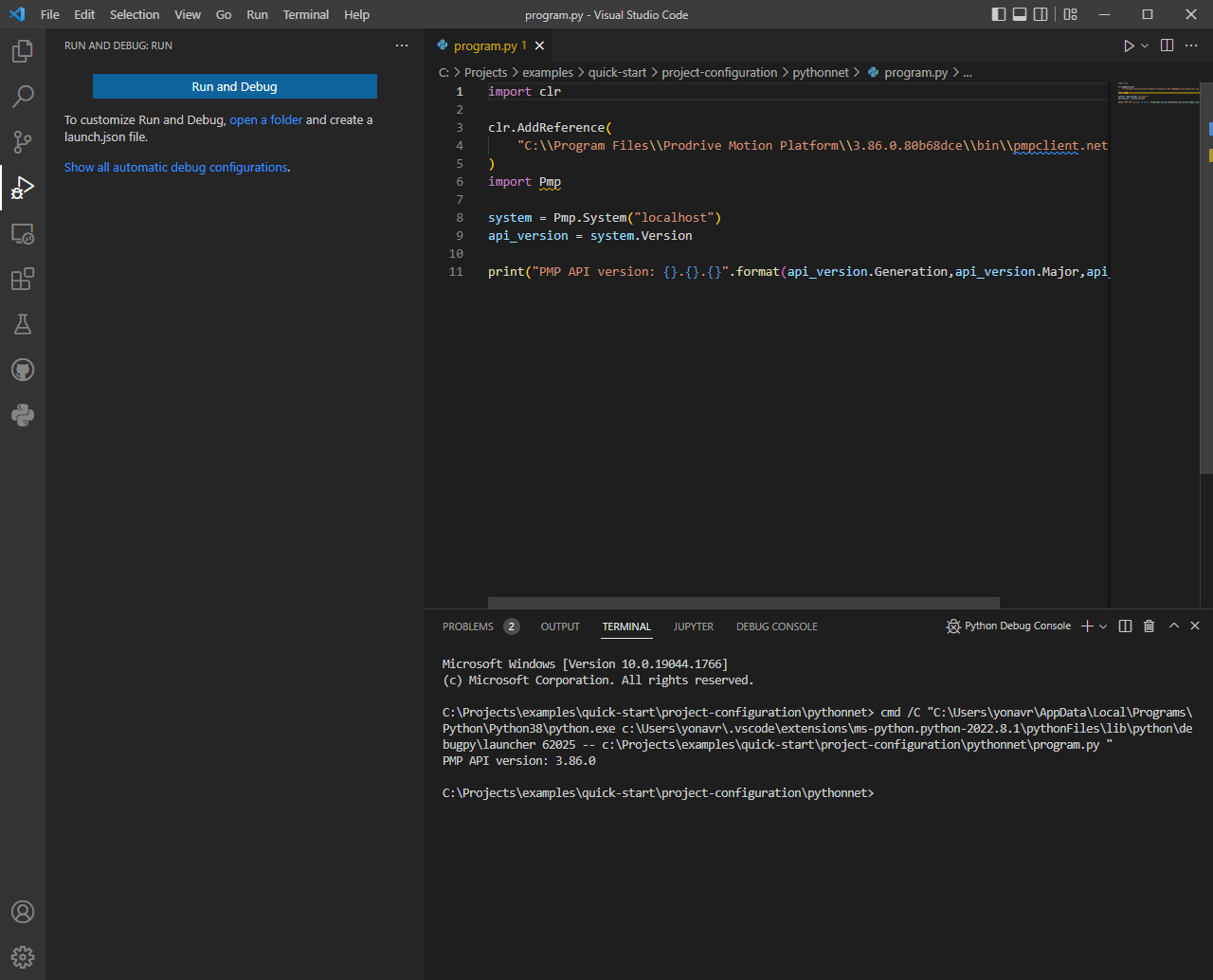
API version¶
A guide for creating a simulated system using Python.NET can be found in the next Create a simulator Quick start chapter.