C++¶
This guide provides step-by-step instructions on how to configure a project to start developing with the PMP C++ API.
During this guide you will learn how to:
Create a C++ console application in Microsoft Visual Studio.
Include the PMP C++ API in the project configuration.
Requirements¶
The PMP C++ API requires a compiler that supports at least C++17. The API is functionally verified with the following compilers:
MSVC++ v142 (Visual Studio 2019)
GCC 10.3
Project configuration¶
During this quick start guide, the 2019 version of Visual Studio is used. However, newer versions of Visual Studio can be used as well.
Create project¶
Open Visual Studio and select Create a new project:
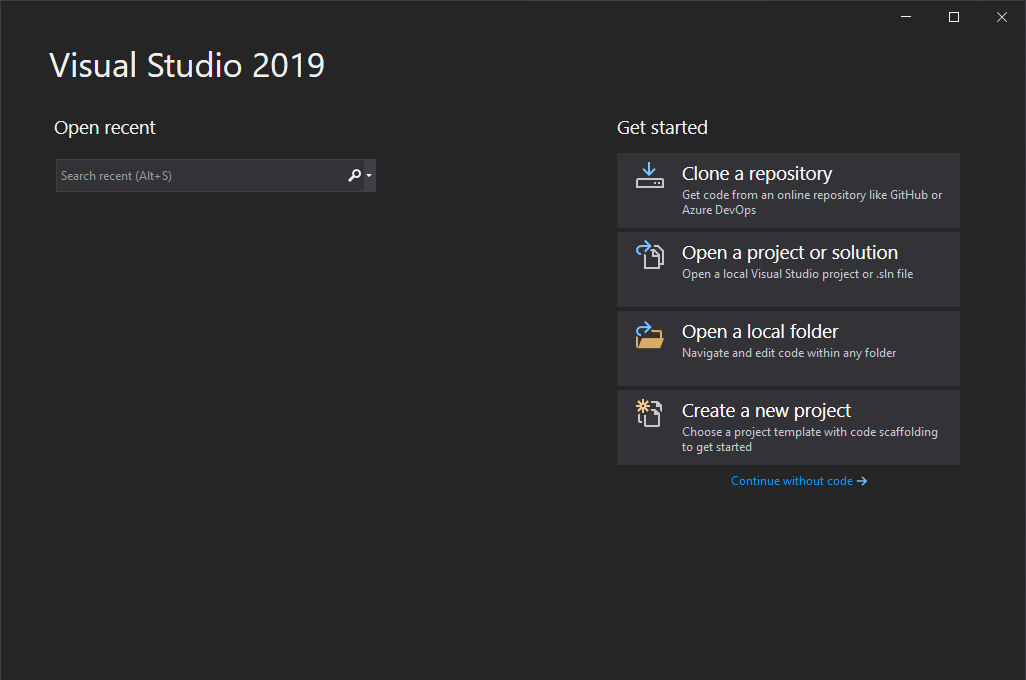
Create a project in Visual Studio¶
Select a C++ Console App, and press Next:
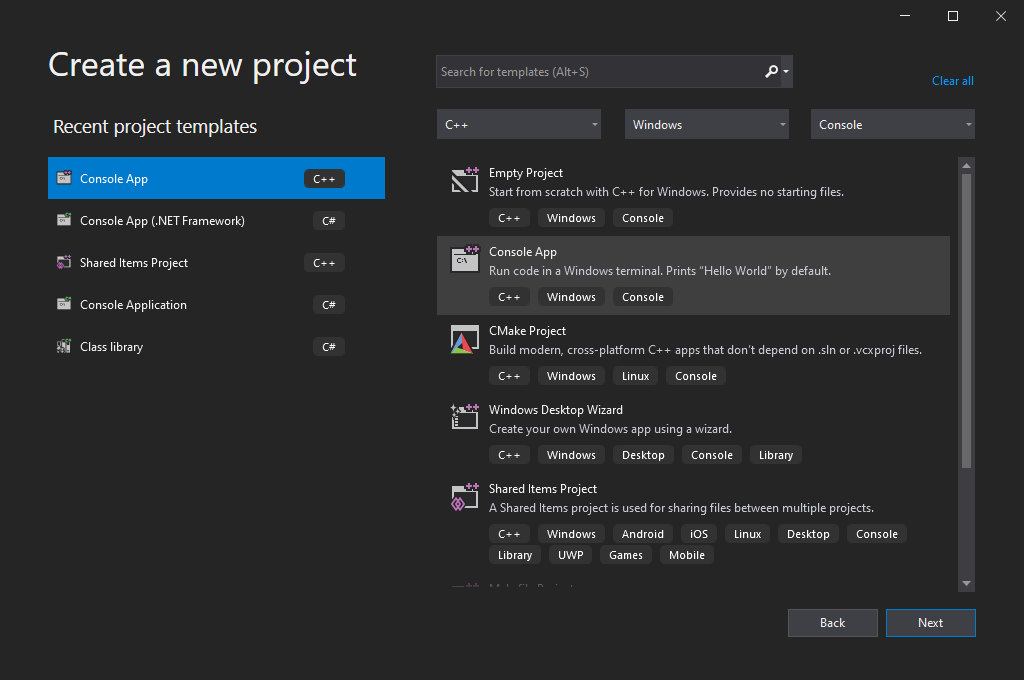
Select C++ console application¶
Select Project name and Location, and press Create:
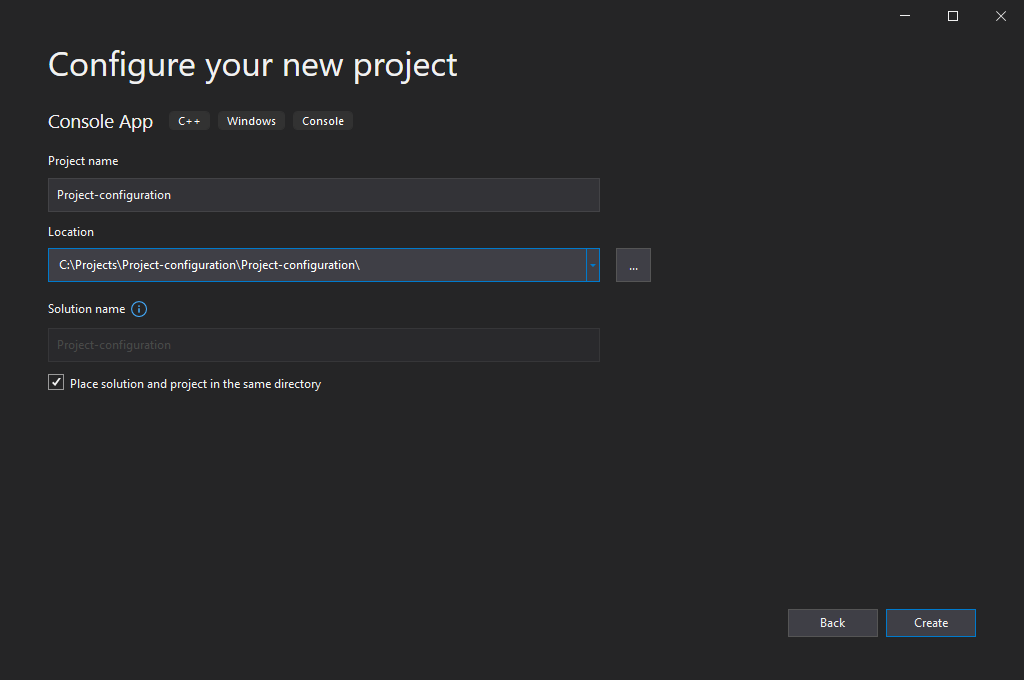
Select project name and directory¶
Configure solution¶
Now that the console application has been created, let us configure the solution. The default console project generated by Visual Studio contains build targets for the Win32 and x64 platforms. The PMP API is only available in 64-bit, so the x86 and Win32 targets should be removed from the solution.
Right-click on the solution name and select Properties.
Go to
.Click on Configuration Manager.
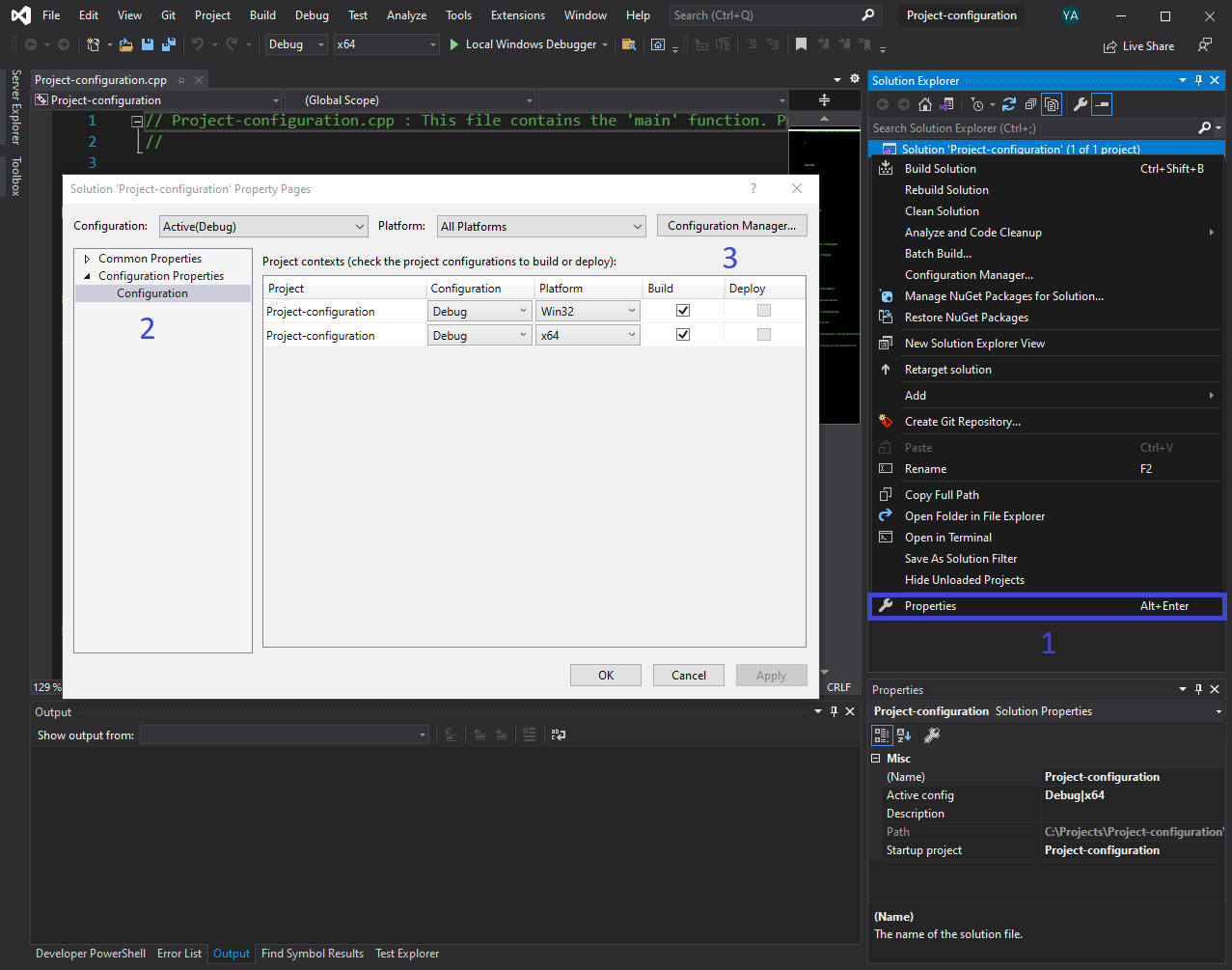
Configure project solution¶
Remove the x86 platform as follows:
In Active solution platform:, select Edit.
Select x86 and remove it from the list.
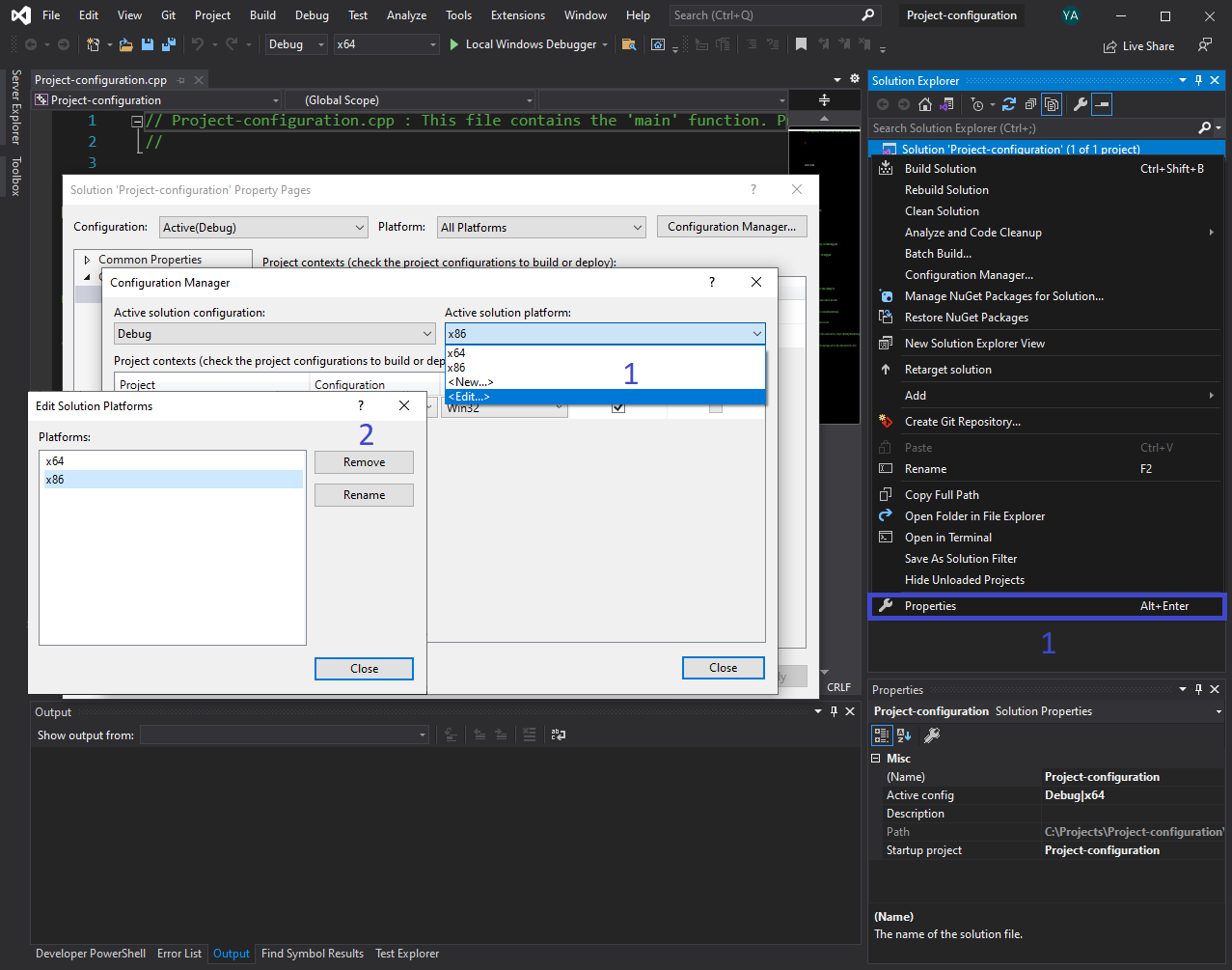
Configure project solution - remove x86 configuration¶
Remove the Win32 platform as follows:
In Platform column, select Edit.
Select Win32 and remove it from the list.
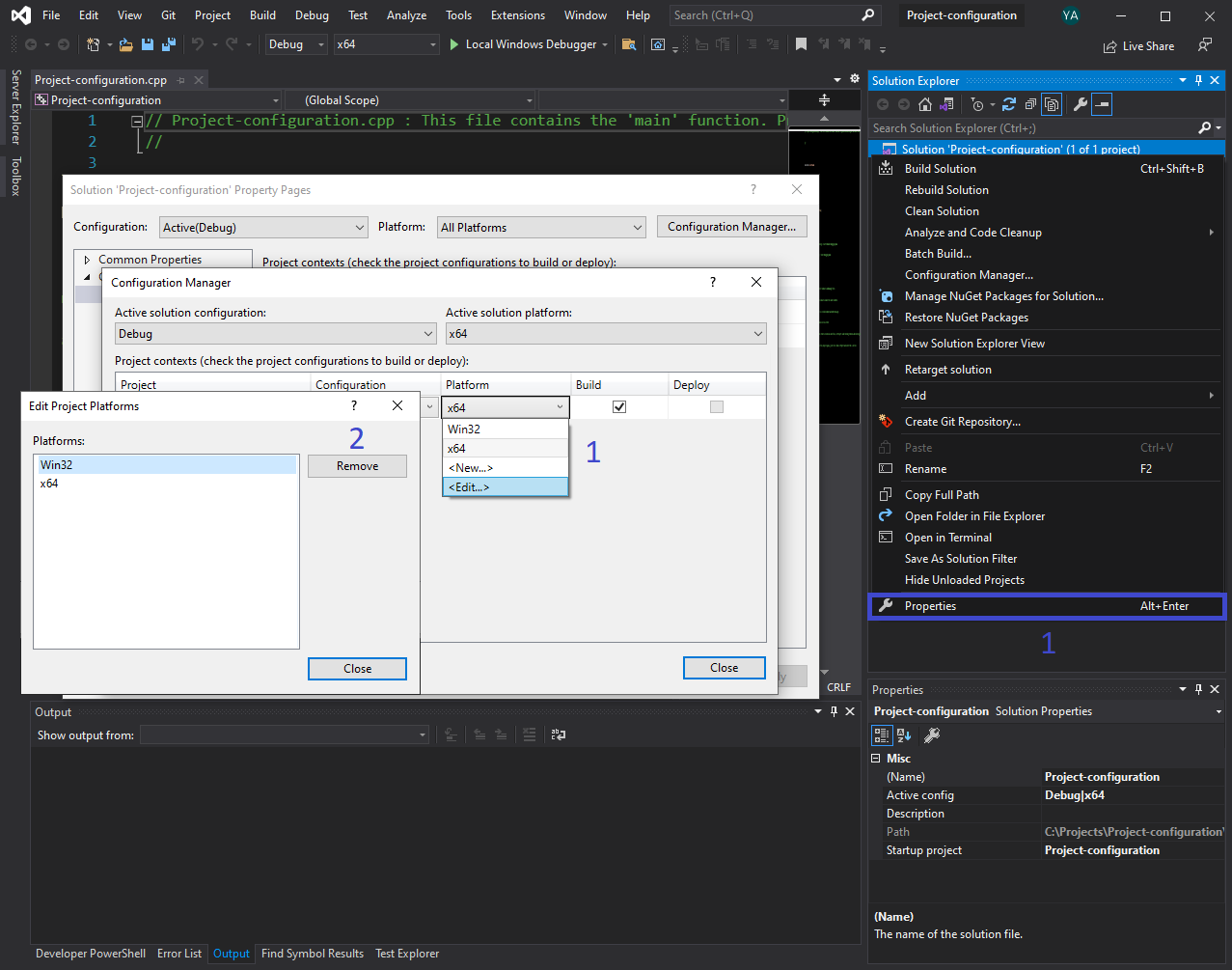
Configure project solution - remove Win32 platform¶
Configure project¶
Now that the solution is configured, let us configure the project settings.
In the Solution Explorer, right click on the project name and select Properties.
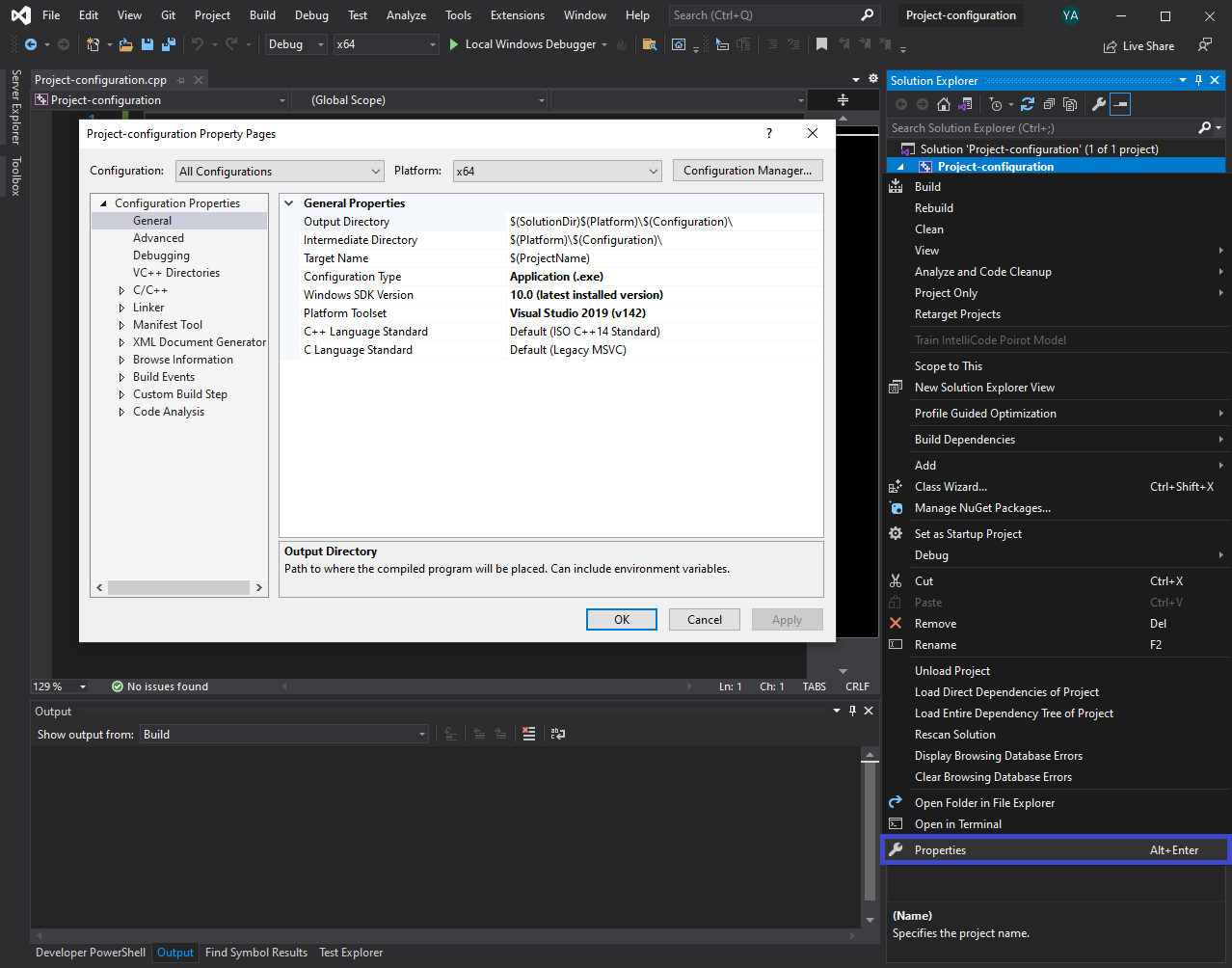
Project setting configuration¶
General¶
Go to
and make sure All Configurations is selected in the Configuration tab.For C++ Language Standard use ISO C++ 17 Standard or higher. This is required to use some of the PMP features.
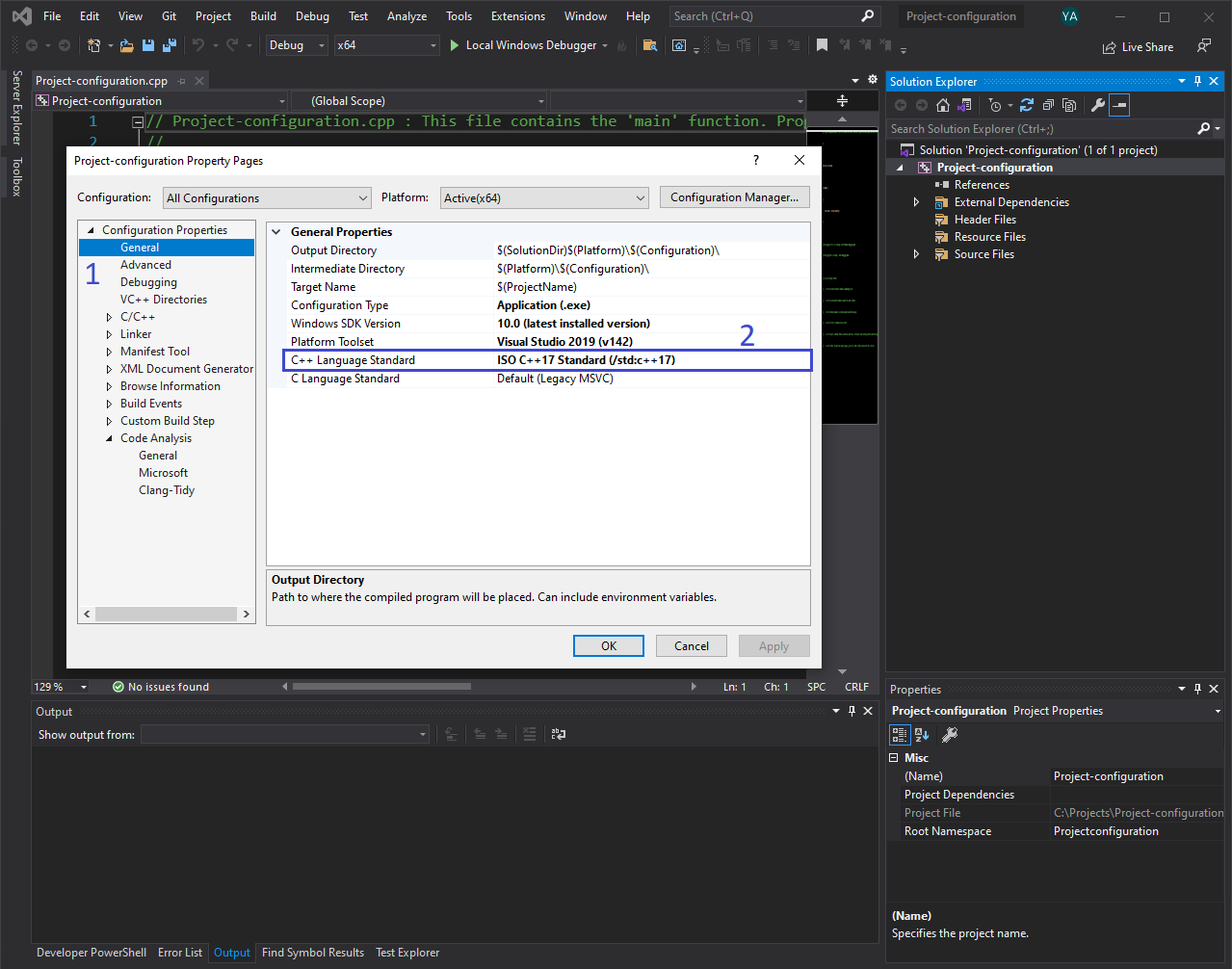
Configure c++ language standard¶
Advanced¶
Go to
.In Configuration:, select Debug.
Change Use Debug Libraries to Yes.
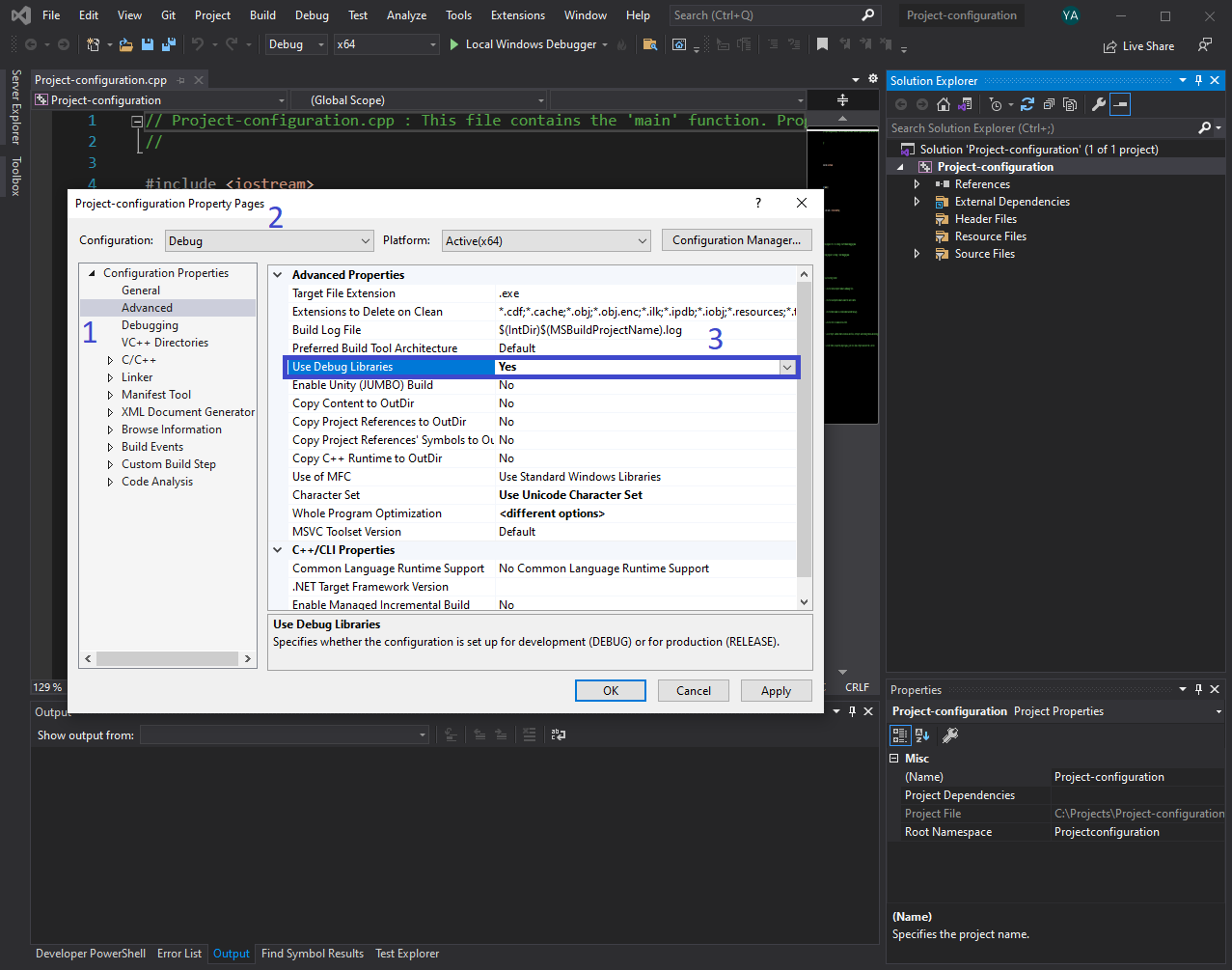
Configure debug libraries¶
C/C++¶
Go to
and make sure All Configurations is selected in the Configuration tab.Add the path to the included pmp header files, which are provided with PMP installation, to Additional Include Directories.
C:\Program Files\Prodrive Motion Platform\96.5.0.4e561701\include;%(AdditionalIncludeDirectories)
Note
Make sure to specify the full PMP version in the above path.
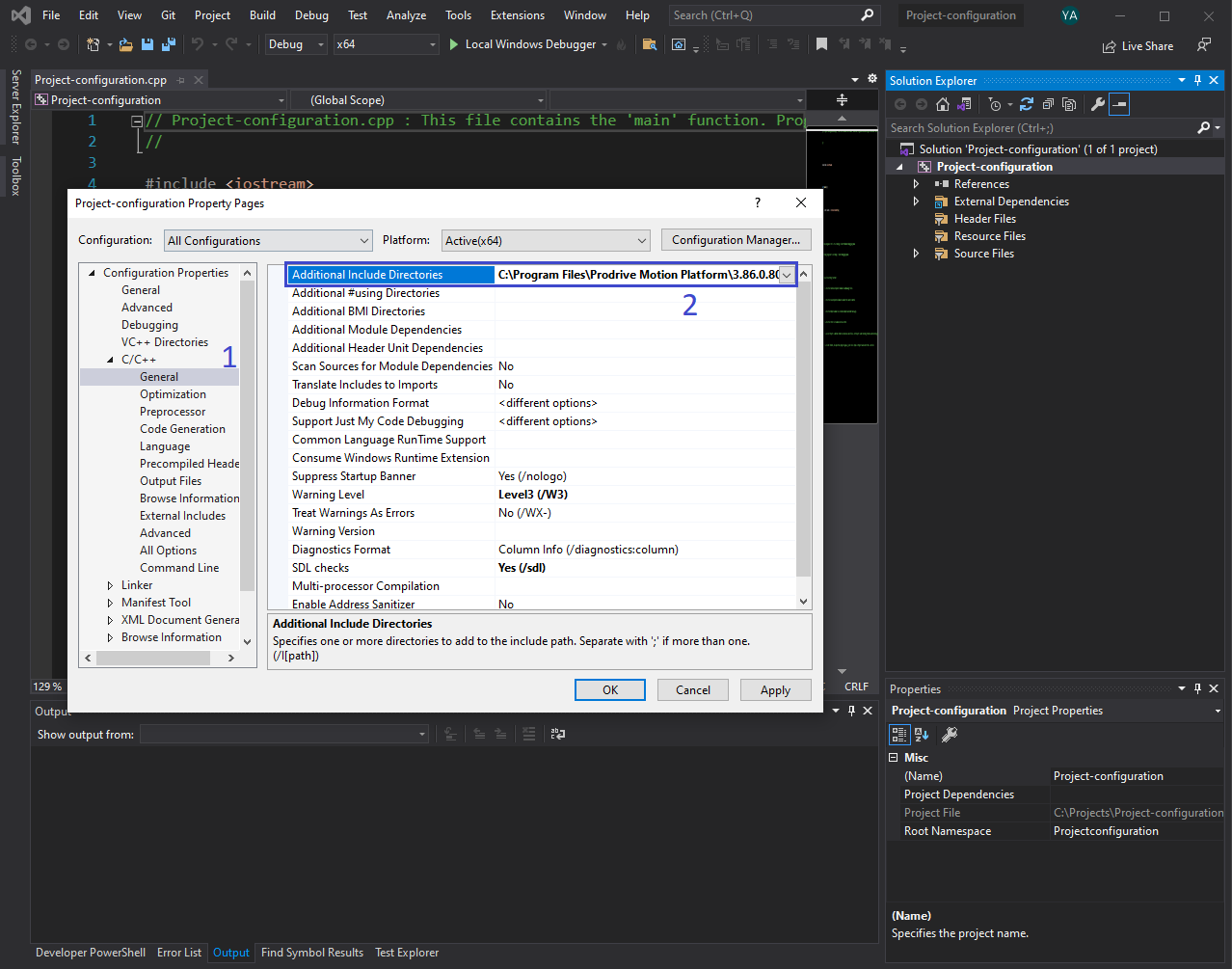
Configure included PMP libraries¶
The PMP API contains a large number of addressable sections.
/bigobj
is used to increase the number of sections that an object file can contain.
Therefore, it is required to configure /bigobj
for the project.
Go to
and make sure All Configurations is selected in the Configuration tab.In the Additional Options, add
/bigobj
.
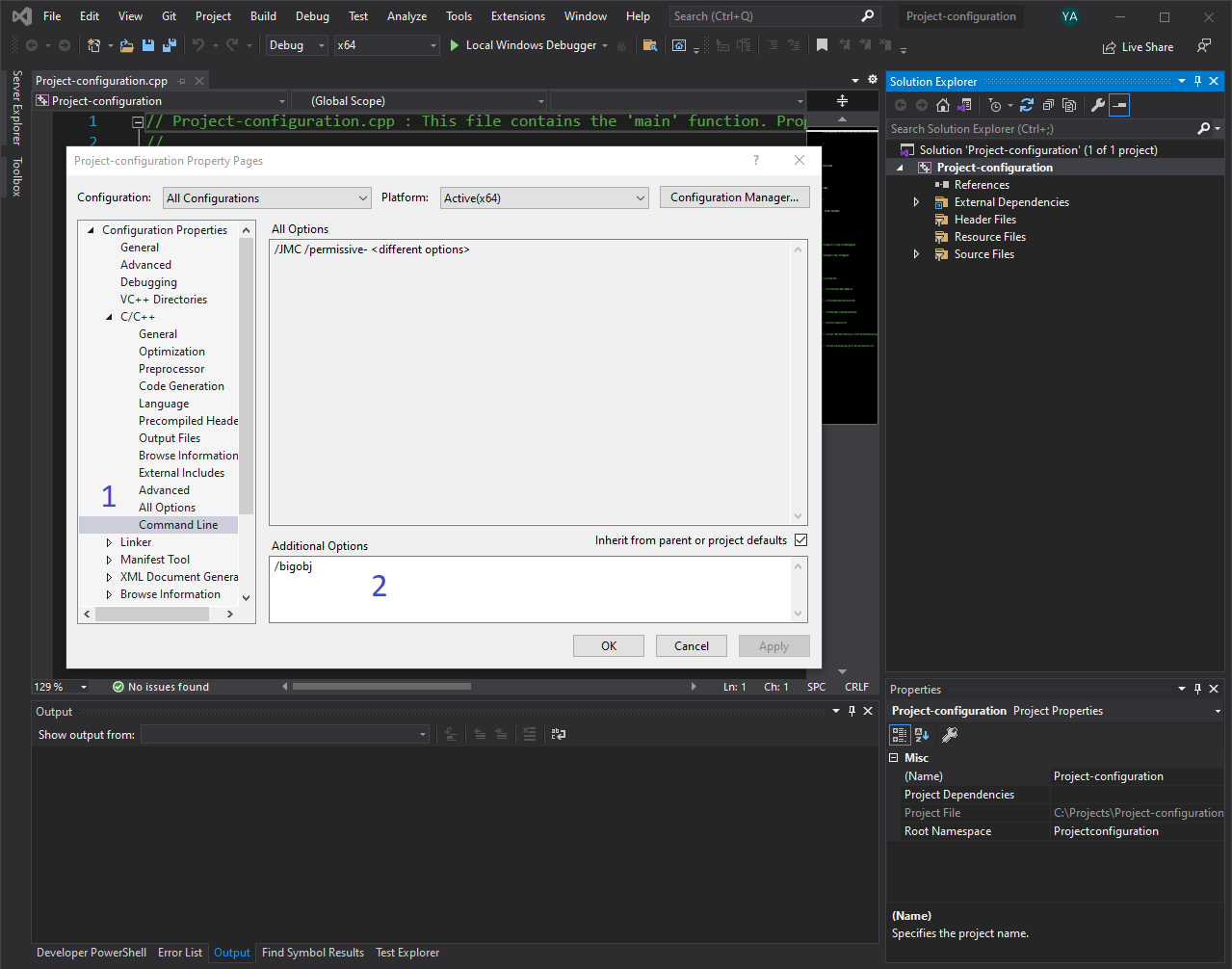
Configure Visual studio for big objects files¶
Linker¶
Now let us add the PMP static library pmpclient.lib
, which is provided with the PMP installation.
Go to
and make sure All Configurations is selected in the Configuration tab.In the Additional Library Directories, add the path to the
pmpclient.lib
as follows:C:\Program Files\Prodrive Motion Platform\96.5.0.4e561701\bin\lib;%(AdditionalLibraryDirectories)
Note
Make sure to specify the full PMP version in the above path.
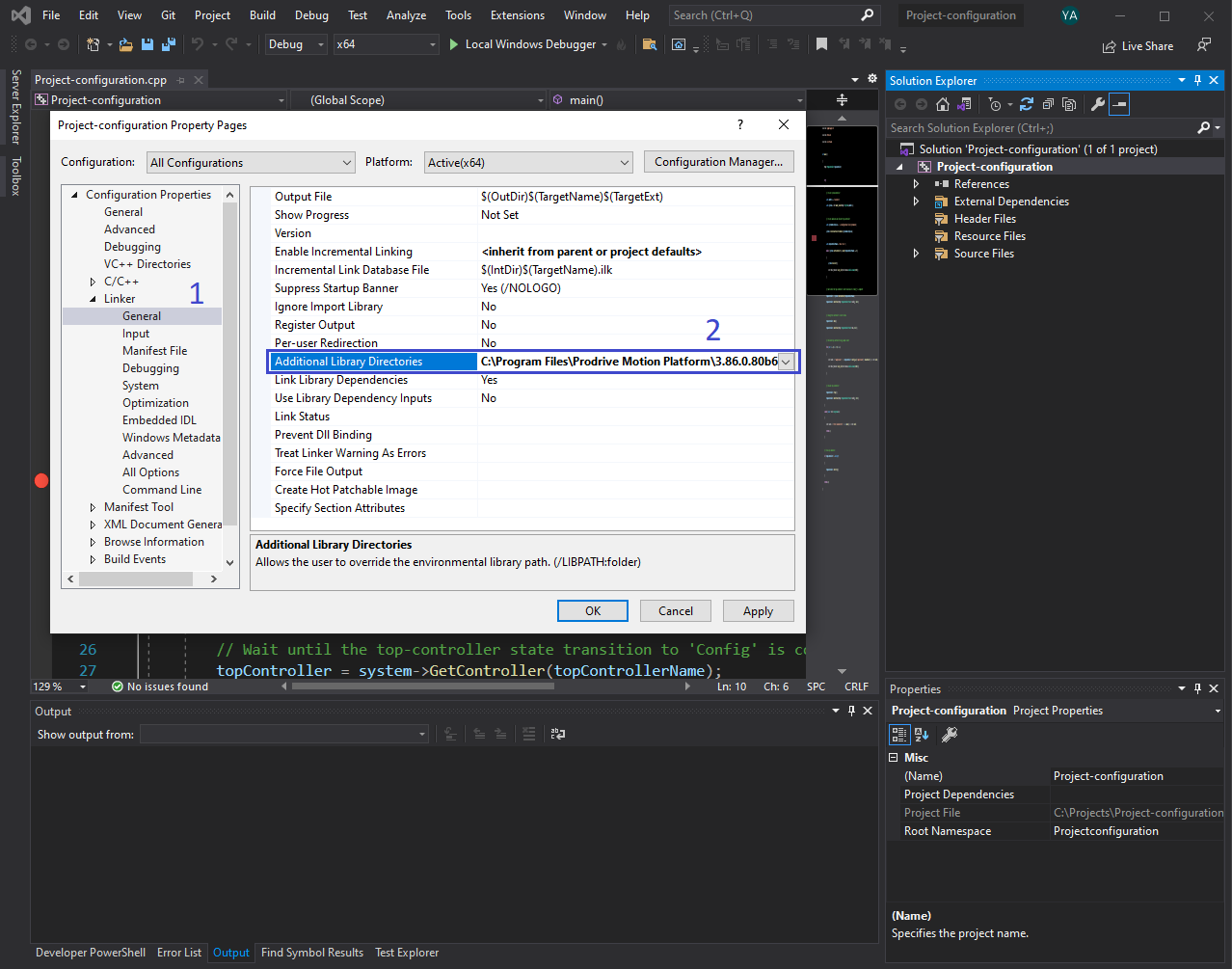
Configure linking properties¶
Go to
and make sure All Configurations is selected in the Configuration tab.In the Additional Dependencies, add the
pmpclient.lib
file as follows:pmpclient.lib;%(AdditionalDependencies)
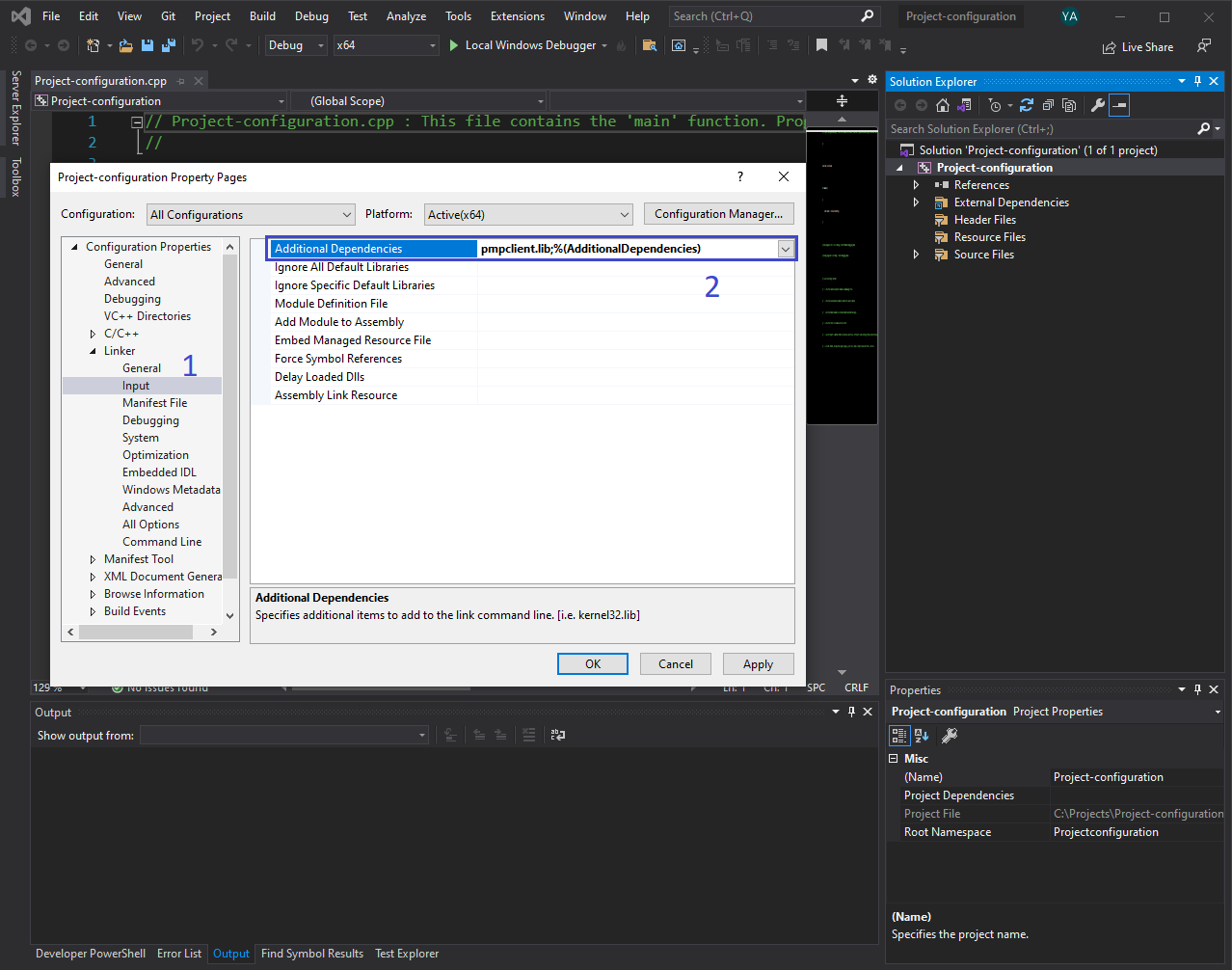
Configure additional dependencies¶
Build Events¶
The PMP C++ API depends on the pmpclient.dll
library.
To run our console application it needs to be able to find this library.
We will configure a post-build event to copy the file to the output folder.
Go to
and make sure All Configurations is selected in the Configuration tab.In the Command Line, add the following command:
copy /y "C:\Program Files\Prodrive Motion Platform\96.5.0.4e561701\bin\pmpclient.dll" "$(OutDir)"
Note
Make sure to specify the full PMP version in the above path.
The command copies the
pmpclient.dll
from the installation bin directory to the output folder.
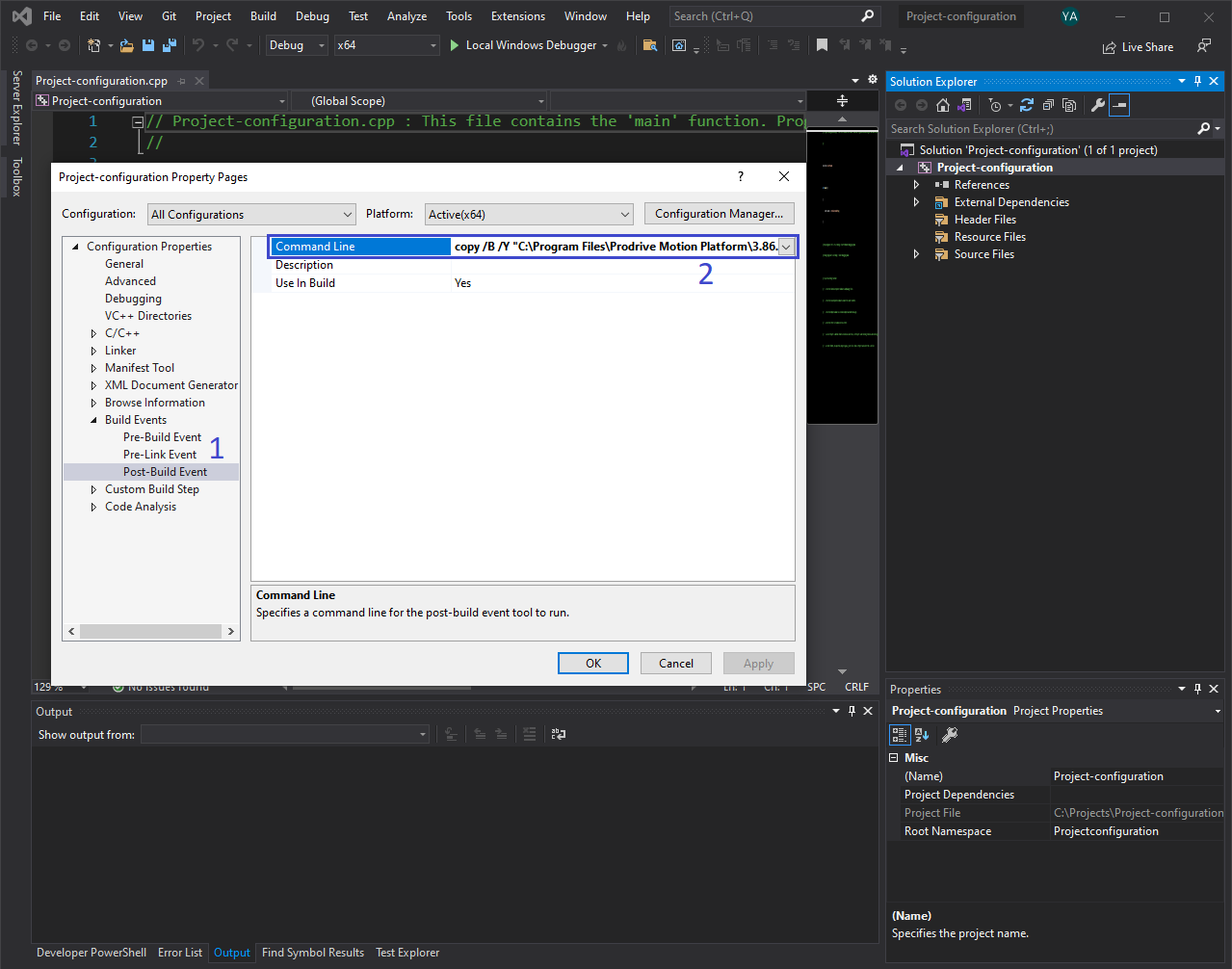
Configure post-build output¶
PMP Wrapper Class¶
The pmpwrapper.cpp
is a C++ wrapper that provides an object-oriented interface
Unlike the pmpclient.dll
, the C++ wrapper is compiled as part of the console application.
The file can be found in the installation src
directory.
Right click on
.Select the
pmpwrapper.cpp
file as follows:C:\Program Files\Prodrive Motion Platform\96.5.0.4e561701\src\pmpwrapper.cpp
Note
Make sure to specify the full PMP version in the above path.
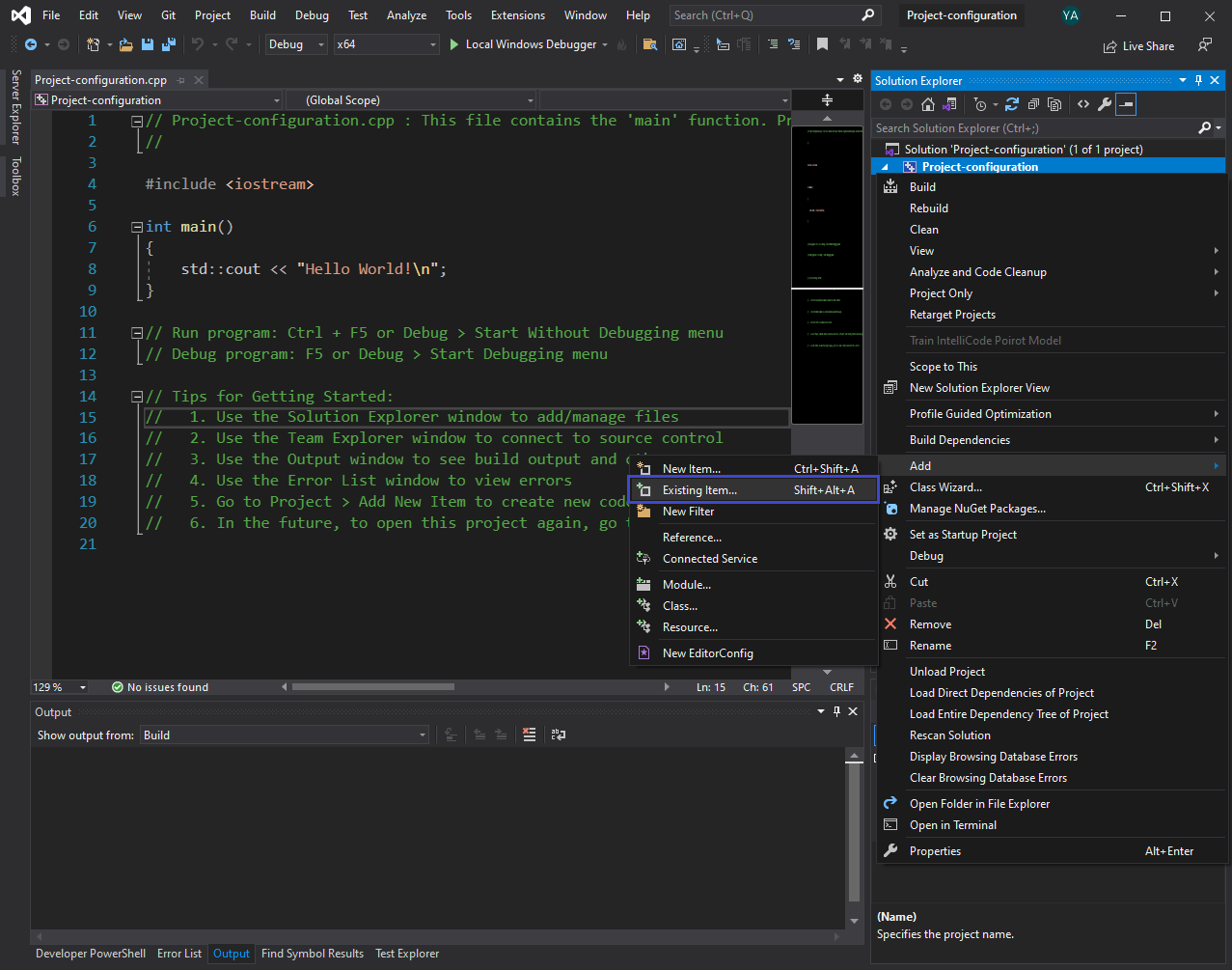
Add pmpwrapper class to a project¶
The pmpwrapper.cpp
shall appear in the solution tree:
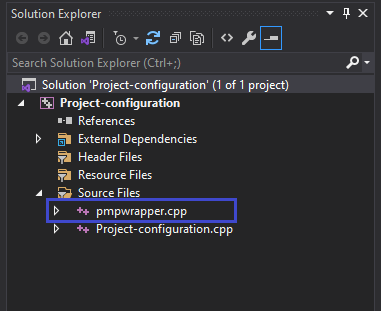
Visual Studio solution tree¶
Note
When your project has the Precompiled Headers option enabled (pmpwrapper.cpp
to include the precompiled header file as first include in pmpwrapper.cpp
.
Example¶
Now that the project is fully configured, a PMP system instance can be created. To verify the system is functioning we can for example get the the API version of the system as shown in the following code:
1 2 3 4 5 6 7 8 9 10 11 12 13 | #include "pmpwrapper.h"
#include <iostream>
int main()
{
auto system = std::make_shared<Pmp::CSystem>("localhost");
auto apiVersion = system->GetVersion();
std::cout << "PMP API version: " << apiVersion.Major << "." << apiVersion.Minor << "." << apiVersion.Patch << std::endl;
return 0;
}
|
Click on Local Windows Debugger or press Ctrl-F5 to start your program.
The API version is displayed in a console and should look similar to:
PMP API version: 96.5.0 C:\your\path\to\Project-configuration\bin\x64\Debug\Project-configuration.exe (process 10284) exited with code 0. Press any key to close this window . . .
A guide for creating a simulated system using C++ can be found in the next Create a simulator Quick start chapter.