MATLAB .NET¶
MATLAB can be used to interact with the PMP system. The easiest way to interface with the PMP API using MATLAB is to use the .NET integration in MATLAB and interface with the .NET PMP API.
During this guide you will learn how to:
Setup your MATLAB environment.
Interface with PMP API using the MATLAB interface to .NET.
Requirements¶
The PMP C# API requires a .NET implementation that supports .NET Standard 2.0.
To determine if your system has the supported framework, use the NET.isNETSupported
command within MATLAB.
>> NET.isNETSupported
ans =
1
The PMP API is only available in 64-bit and a 64-bit version of MATLAB is required to interface with the API. To verify you are using a 64-bit version of MATLAB, use the following command in the MATLAB command window:
>> computer('arch')
ans =
win64
Project configuration¶
To start you program, create a new MATLAB script.
Add .NET assembly¶
It is sufficient to add the pmpclient.net.dll to MATLAB, the pmpclient.dll will automatically be called when placed in the same folder.
The .NET assembly can be added via the NET.addAssembly
command:
pmpclient = NET.addAssembly('C:\\Program Files\\Prodrive Motion Platform\\96.5.0.4e561701\\bin\\pmpclient.net.dll');
The assembly object contains information about available properties.
>> pmpclient
pmpclient =
NET.Assembly handle
Package: NET
Properties for class NET.Assembly:
AssemblyHandle
Classes
Structures
Enums
GenericTypes
Interfaces
Delegates
To obtain the corresponding methods, properties of an interface, or enumeration members of an enumeration, see General MATLAB .NET Interfacing.
Example¶
Now that the project is fully configured, a PMP system instance can be created. To verify the system is functioning we can for example get the the API version of the system as shown in the following code:
1 2 3 4 5 6 | pmpclient = NET.addAssembly('C:\\Program Files\\Prodrive Motion Platform\\%PMP_VERSION%\\bin\\pmpclient.net.dll');
system = Pmp.System('localhost');
apiVersion = system.Version;
fprintf('PMP API version: %d.%d.%d\n', apiVersion.Major, apiVersion.Minor, apiVersion.Patch)
|
From the main menu tab select
or press F5.The API version is displayed in the command window.
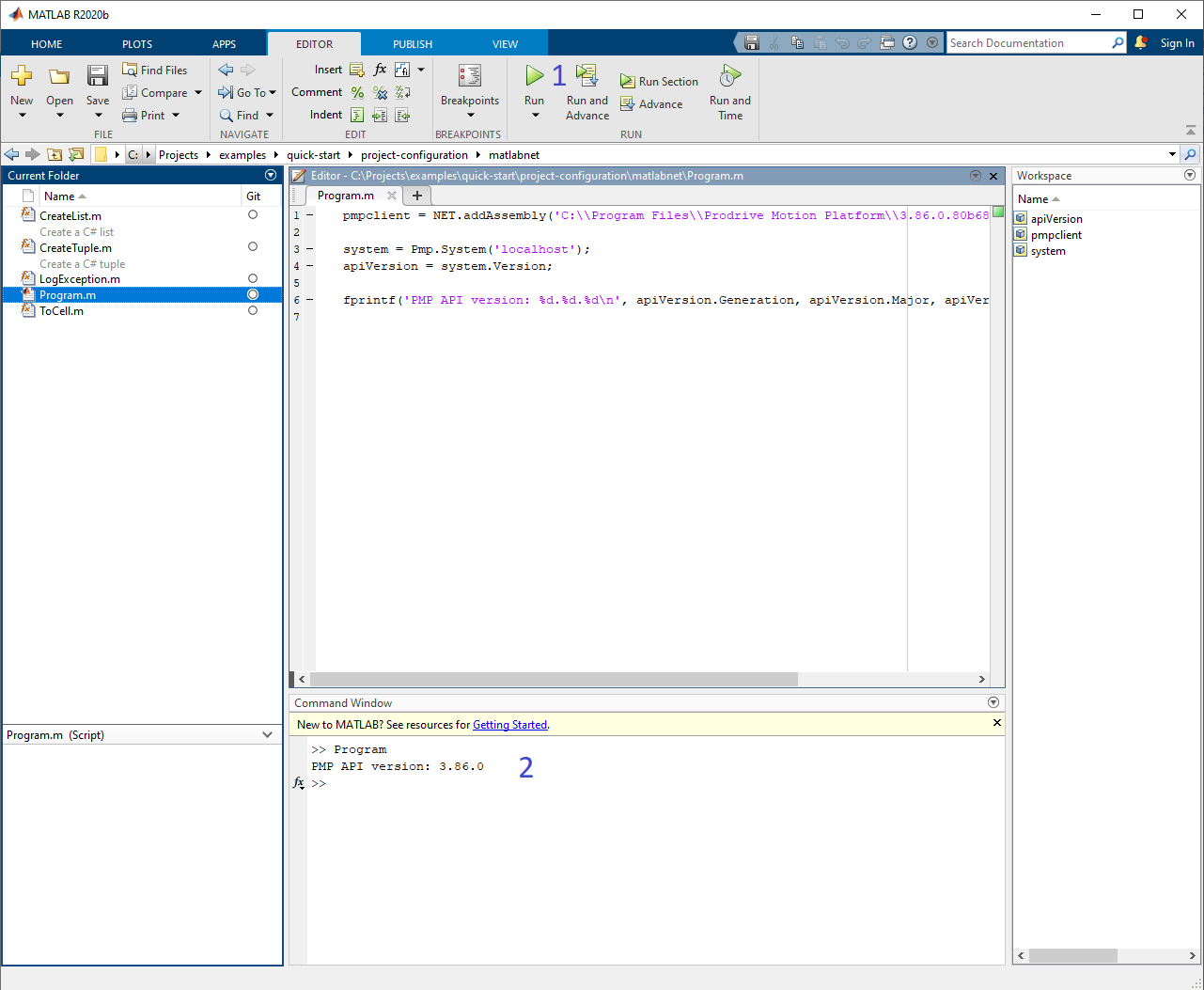
API version¶
Limitations¶
The MATLAB interface to .NET has several limitations:
The assembly cannot be unloaded, to unload the PMP client assembly MATLAB needs to be restarted.
MATLAB is a single-threaded application and does not support direct access to threads without the Parallel Computing Toolbox. Therefore, be careful when using callbacks, as this can cause deadlocks in your application.
For more limitations to .NET support see https://www.mathworks.com/help/matlab/matlab_external/limitations-to-net-support.html.
A guide for creating a simulated system using MATLAB .NET can be found in the next Create a simulator Quick start chapter.