Simulink code generation¶
This guide describes how to create a Processing block binary file from on a Simulink model that can be loaded into a (simulated) PMP motion controller or PMP EtherCAT SubDevice. The Deployment guide explains how to upload this binary file on a PMP device or simulator. Simulink knowledge is a prerequisite.
During this guide you will learn how to:
Configure Simulink to support code generation for motion controllers and EtherCAT SubDevices.
Create a simple Simulink model.
Use code generation to build a binary file that can be deployed on a (simulated) PMP motion controller or PMP EtherCAT SubDevice.
Introduction¶
PMP provides a MATLAB / Simulink toolbox that build a binary file from a Simulink model, that can be uploaded onto a PMP motion controller or EtherCAT SubDevice. This makes it possible to implement custom functionality that is executed in real-time. The toolbox uses Simulink Coder to generate code from the Simulink model, and adds PMP-specific wrapper functionality that allows the motion controller to interact with the generated code. From the Simulink model it is possible to create signals, inputs, updatables, events and filters in PMP. Typical use cases are control loops, compensation algorithms, coordinate transforms and custom state handling.
Since control design is often performed in MATLAB / Simulink, Simulink code generation fits well into a typical development process:
A control engineer sets up a control loop in Simulink with a plant model and a controller implementation. This simulation is a great tool to get insight in the control system and try new ideas.
Using Simulink code generation both the controller implementation and the plant model are exported. They can then be integrated into a PMP simulator so that also the software integration can be tested.
Once the design is verified on the PMP simulator, it can be deployed to hardware. If improvements need to be made, the Simulink model can easily be updated and re-exported. Optionally the changes can be tested in the Simulink simulation or on the PMP simulator. Changes can be applied on the motion controller without rebooting, so quick design iterations can be made. Therefore a systems / control / application engineer can easily make changes without support of a software engineer.
Simulink code generation control loop customization reference manual explains the code generation process, considerations and supported interfaces in detail.
Prerequisites¶
Before continuing with the user guide, please make sure that the following prerequisites are met:
PMP should be installed. The Complete setup can be used to install all required features, including Simulink code generation support. Alternatively, the Custom setup can be used to save disk space. In this case make sure the sub-feature Simulink toolchain under Control loop customization is selected.
MATLAB installation of a supported version with the following toolboxes installed:
Simulink
MATLAB Coder
Simulink Coder
Installation of a supported compiler.
The Simulink code generation guide project files should be available. These files can be downloaded from Downloads.
Note
A generated binary can be used on both motion controllers and EtherCAT SubDevices. However, for EtherCAT SubDevices the interfaces (i.e. inports, outports, testpoints, filters, LUTs) for processing blocks are predefined in the SubDevice firmware. For each processing block of the PMP EtherCAT SubDevice a Simulink model with the supported interfaces is provided in the product specific installer. This model can be used as starting point. This manual focusses on processing blocks for motion controllers.
Configuration¶
The PMP Simulink installation directory is a sub directory of the PMP installation directory:
C:\Program Files\Prodrive Motion Platform\96.5.0.4e561701\matlab\simulink\
The path needs to be added to the MATLAB path to use the pmp.tlc
file, which is required to generate code.
Open MATLAB.
In the terminal, check if the pmp.tlc
is already added to the path using the following command:
which('pmp.tlc')
If the file’s directory is not found, manually add the directory to the path as follows:
Select Set Path in the Home tab of the ribbon bar.
Click on Add Folder… and select the Simulink directory listed above.
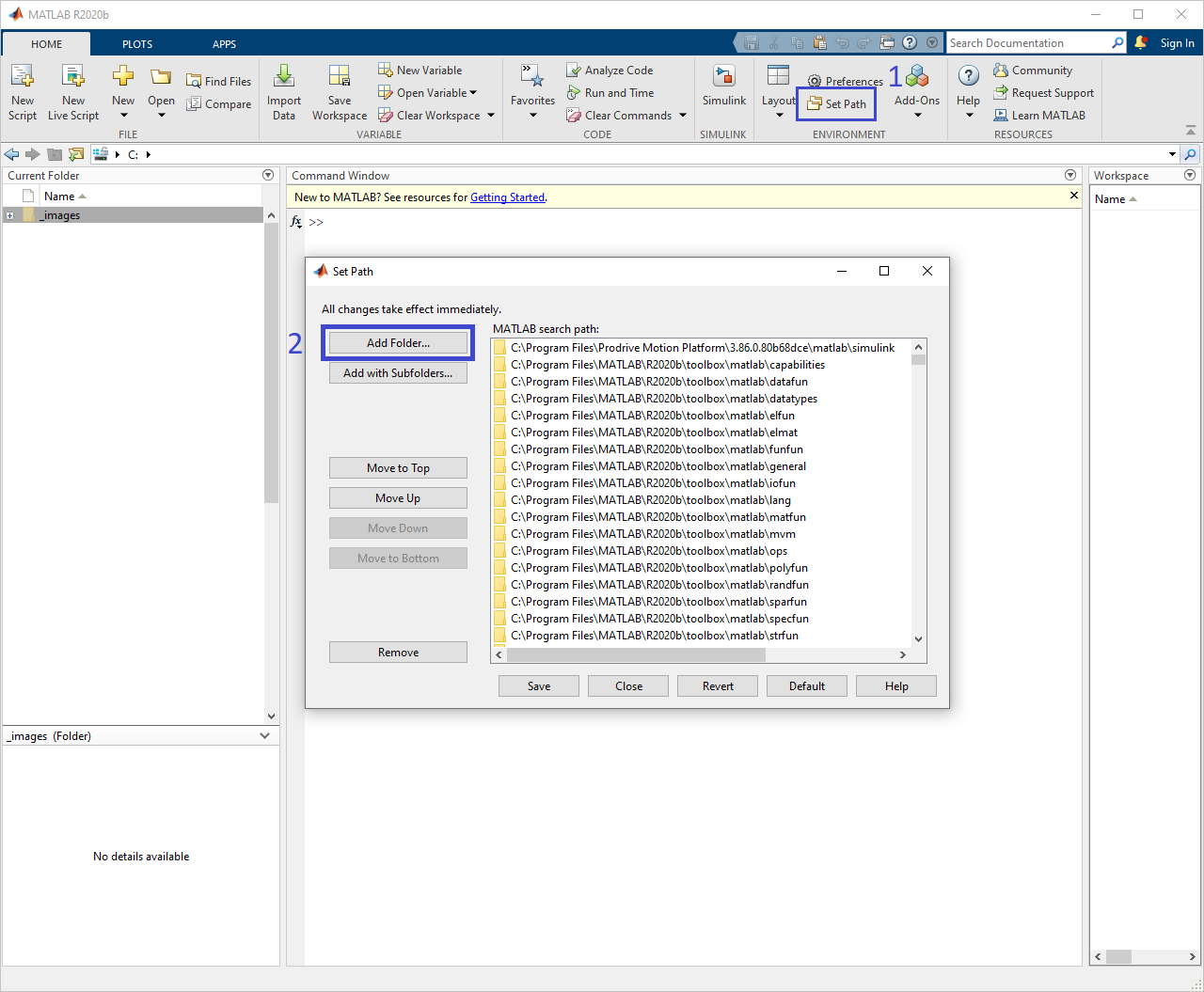
Add PMP Simulink folder to the MATLAB path¶
The PMP libraries are now added to the Simulink library and can be accessed as follows:
Open Simulink in the Home tab of the ribbon bar
Create a Blank Model.
Click on Library Browser to open all libraries.
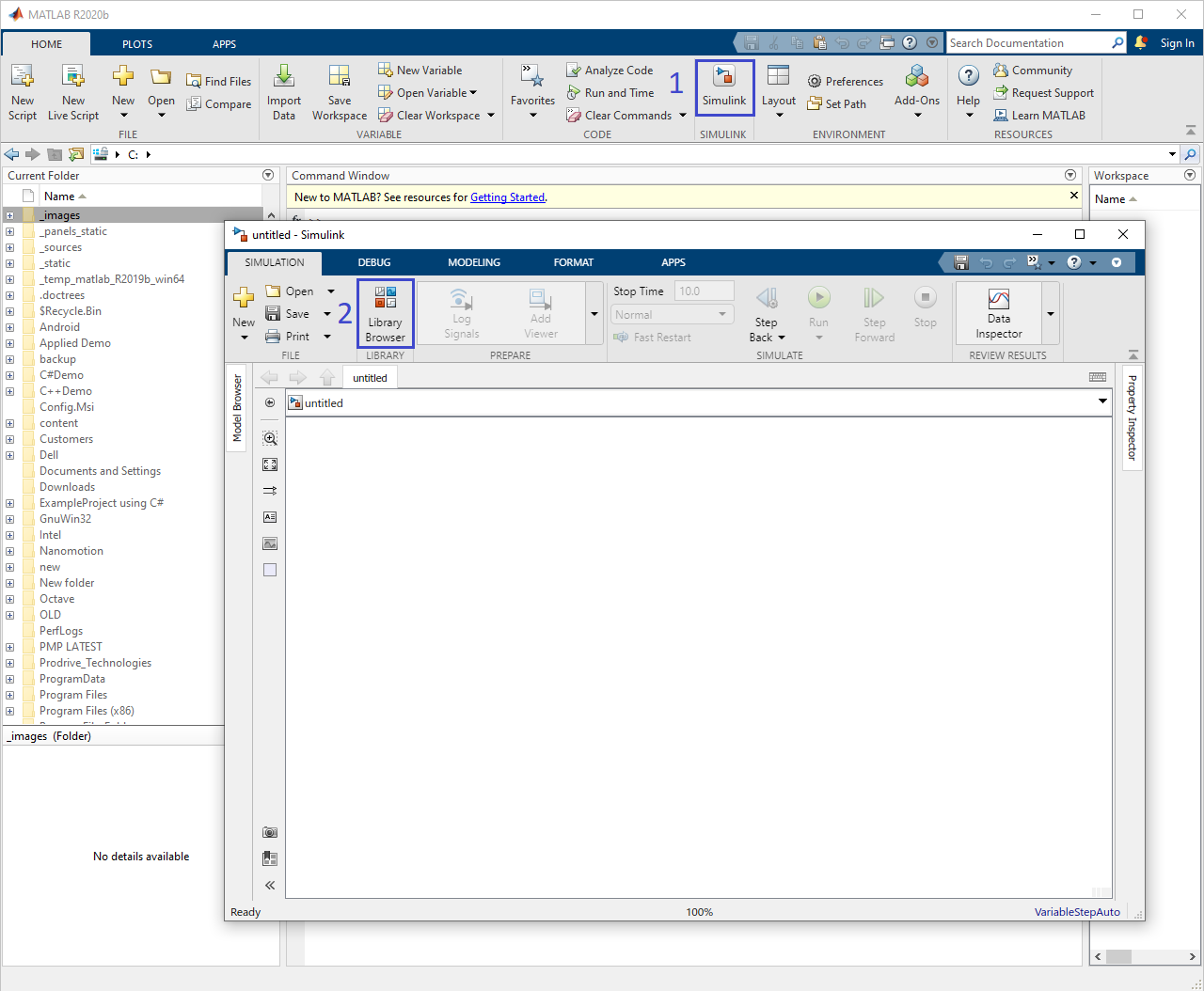
Open Simulink¶
If the Prodrive Motion Platform library is visible the PMP installation directory is correctly recognized by MATLAB.
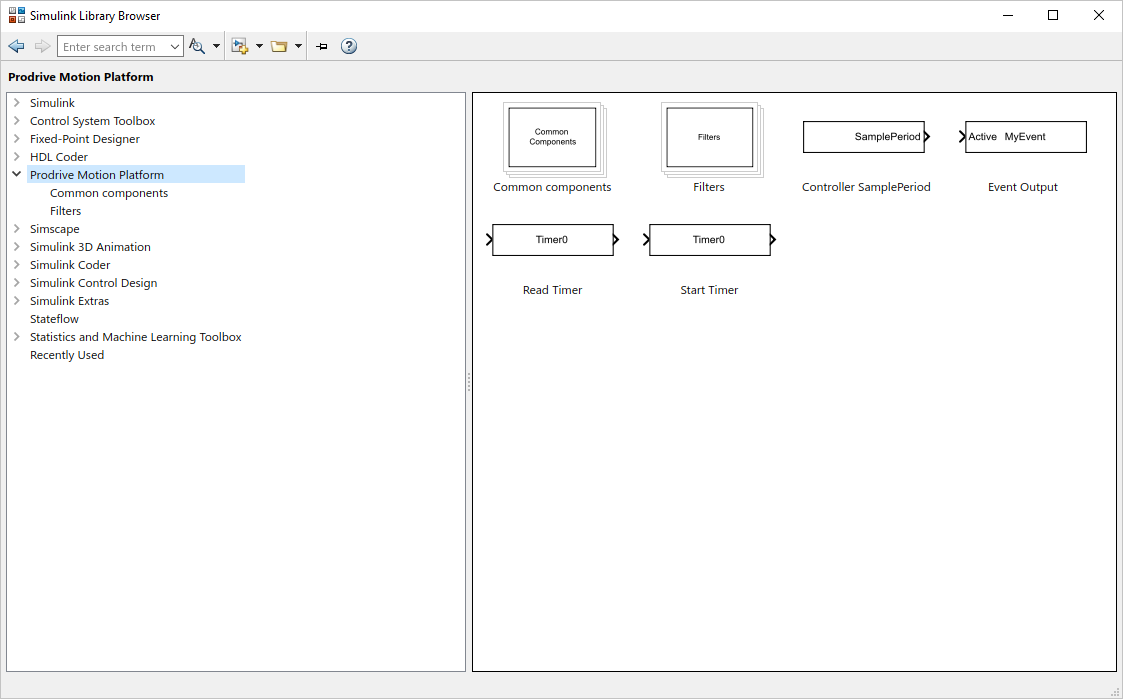
Prodrive Motion Platform library in the library browser¶
Simulink model¶
Create a Simulink model¶
As an example, we will create a feedforward block, which will be connected to a position feedback control in the Deployment guide. The block will take velocity and acceleration setpoint inputs from the trajectory interpolator and calculate the feedforward output.
The feedforward model is created in Simulink as follows:
Create Inport ports that will receive signals DemandVelocity and DemandAcceleration from the trajectory interpolator.
Create Gain blocks with variables: Kfv, Kfc and Kfa and add a Sign block for the Coulomb feedforward.
Create an Add block to sum all values.
Create an Outport port.
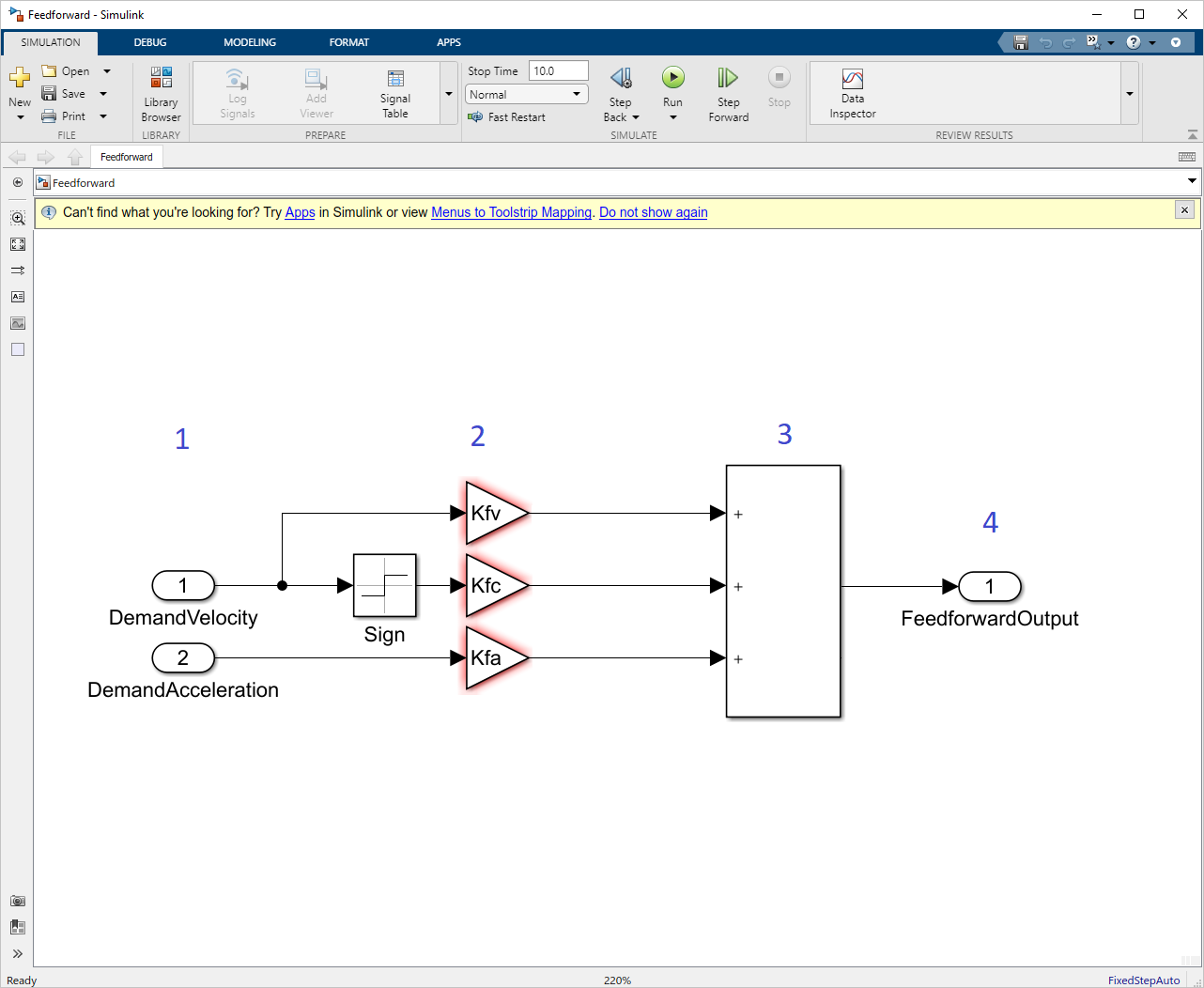
Feedforward model in Simulink¶
Note
The gain variables are marked in red since they have not been defined yet. The definition of variables is discussed later in Parameters.
Mapping to PMP objects¶
Inputs¶
During code generation, all Simulink inports are converted into PMP Input, i.e. the DemandVelocity and DemandAcceleration inports will become PMP inputs. Once the generated code is deployed, these inputs can be connected to signals within PMP. A bus or a vector inport will result in multiple PMP inputs since PMP inputs only support scalar values.
Parameters¶
By default, all Simulink block parameters are fixed during code generation and cannot be changed in PMP. To make parameters tunable in PMP, the parameter can be declared as PmpParameter object in Simulink.
The following MATLAB command should is used to create the PmpParameter Kfv:
Kfv = PmpParameter(min, max, resetValue, dataTypeStr, unit, description);
With the arguments:
Parameter |
Description |
---|---|
min and max |
Used to limit what values are allowed to be configured. An empty argument can be inserted using |
resetValue |
The default value of the parameter. |
dataTypeStr |
Data-type of the parameter (string). Available type are: |
unit |
Unit of the parameter (string). Can be left empty using |
description |
Description of the parameter (string). Can be left empty using |
After code generation, a read/write PMP signal is generated that can be used to modify the value during execution.
Signals¶
Intermediate calculation results in the Simulink model are not visible in the PMP API unless they are marked as a test point. Test points defined in Simulink and outports will automatically appear as read-only signals in PMP and can be traced and monitored.
To create a test point:
Right-click on the route connected to the output of the Kfv gain block and select Properties.
Set the signal name to KfvOutput.
Check the Test point box.
Create a Simulink test point¶
Repeat these steps for the routes connected to the outputs of the Kfc and Kfa gains blocks.
Simulink feedforward with all test points¶
By default, a PMP Signal is generated without metadata (min, max, unit, description). Optionally metadata can be added by specifying a PmpSignal variable in the workspace using the same name as the signal name of the test point or outport.
KfvOutput = PmpSignal(min, max, unit, description);
Where the arguments are:
Parameter |
Description |
---|---|
min and max |
Used to limit what values are allowed to be configured. An empty argument can be inserted using |
unit |
Unit of the parameter (string). Can be left empty using |
description |
Description of the parameter (string). Can be left empty using |
The test point defined in the Simulink model with the name KfvOutput will now appear in PMP as signals with the description, min, max, and unit as specified. The data type of the signal is derived from the model.
Information overlays¶
Before proceeding, check the output data types and sample period of all blocks in the model.
Data types¶
It is important to consider the data types used throughout the model. For this example, we shall use the single type for all inports. The rest of the signals can remain on inherited (auto) and thus automatically defined by Simulink.
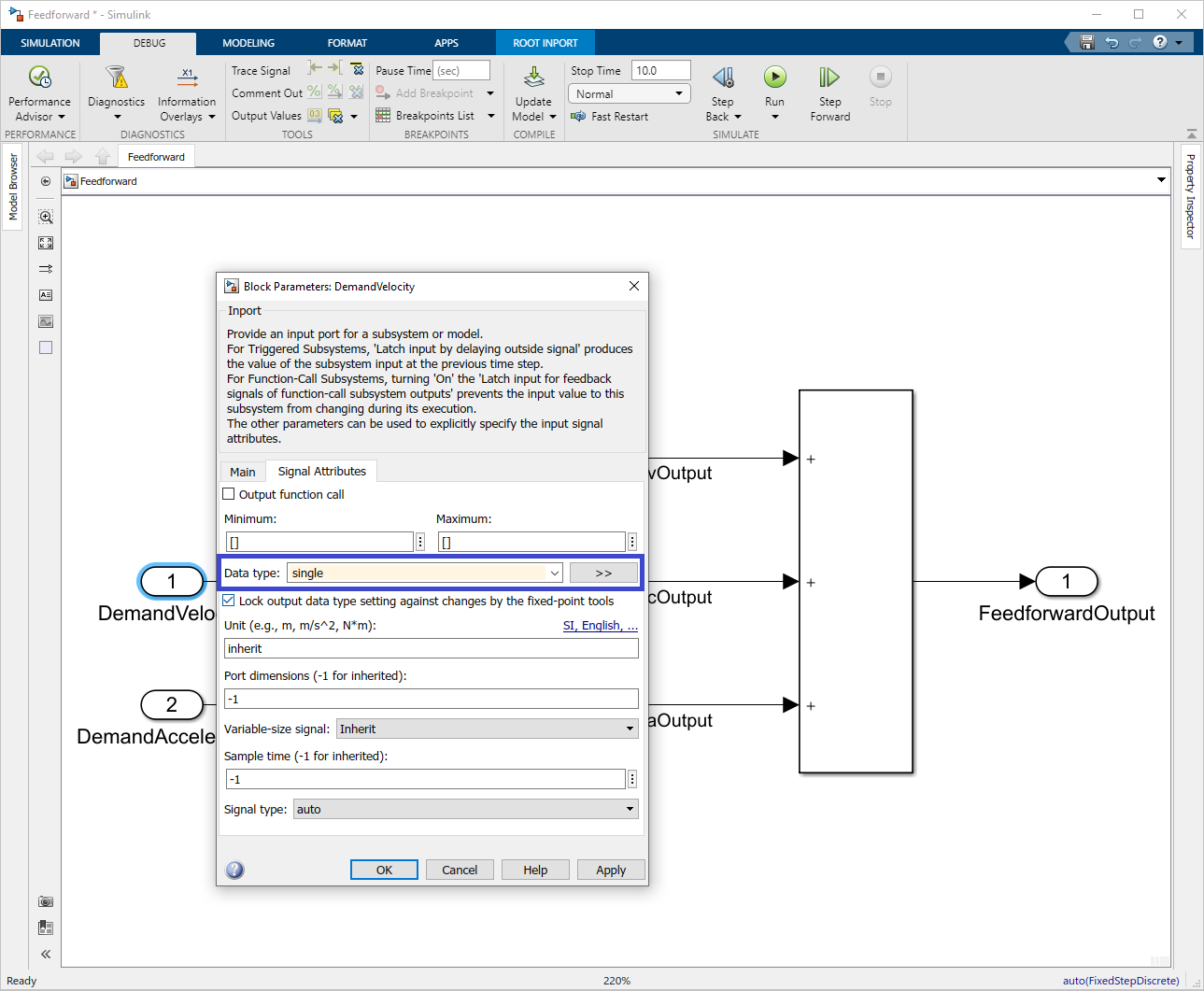
Set signal datatype to single¶
To verify that inheritance went as expected, the data type of each signal can be made visible via
.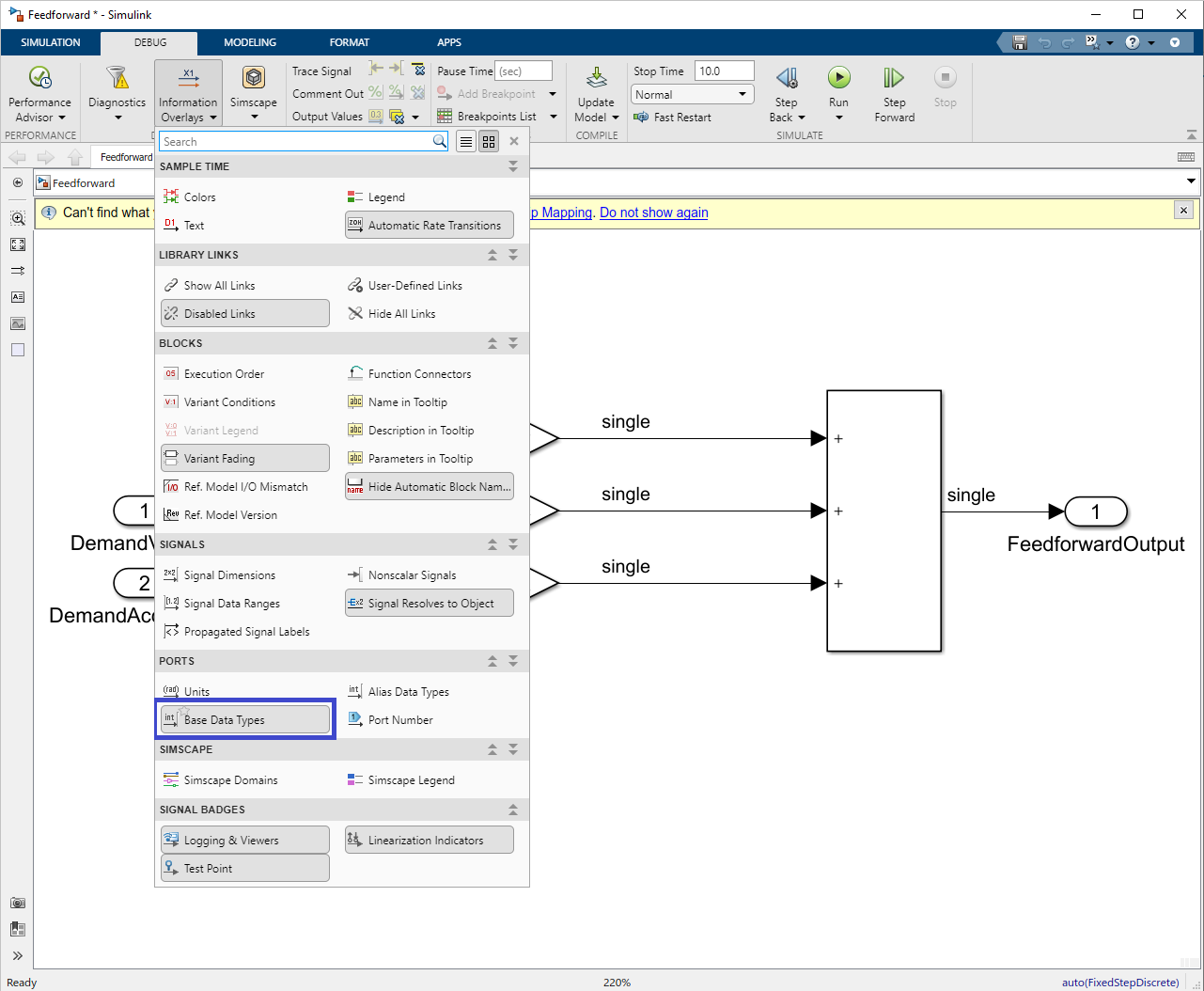
Feedforward model annotated with datatypes¶
Sample period¶
The sample period of the model is set during the code generation procedure, which will be discussed later in this guide. However, to verify that the sample periods are consistent throughout the model, a visual tool can be used.
First we need to define a fixed step solver as follows:
Click on
.In the solver selection, select Fixed-step.
In the solver details set the Fixed-step size (fundamental sample time) to 0.0005 seconds (2kHz).
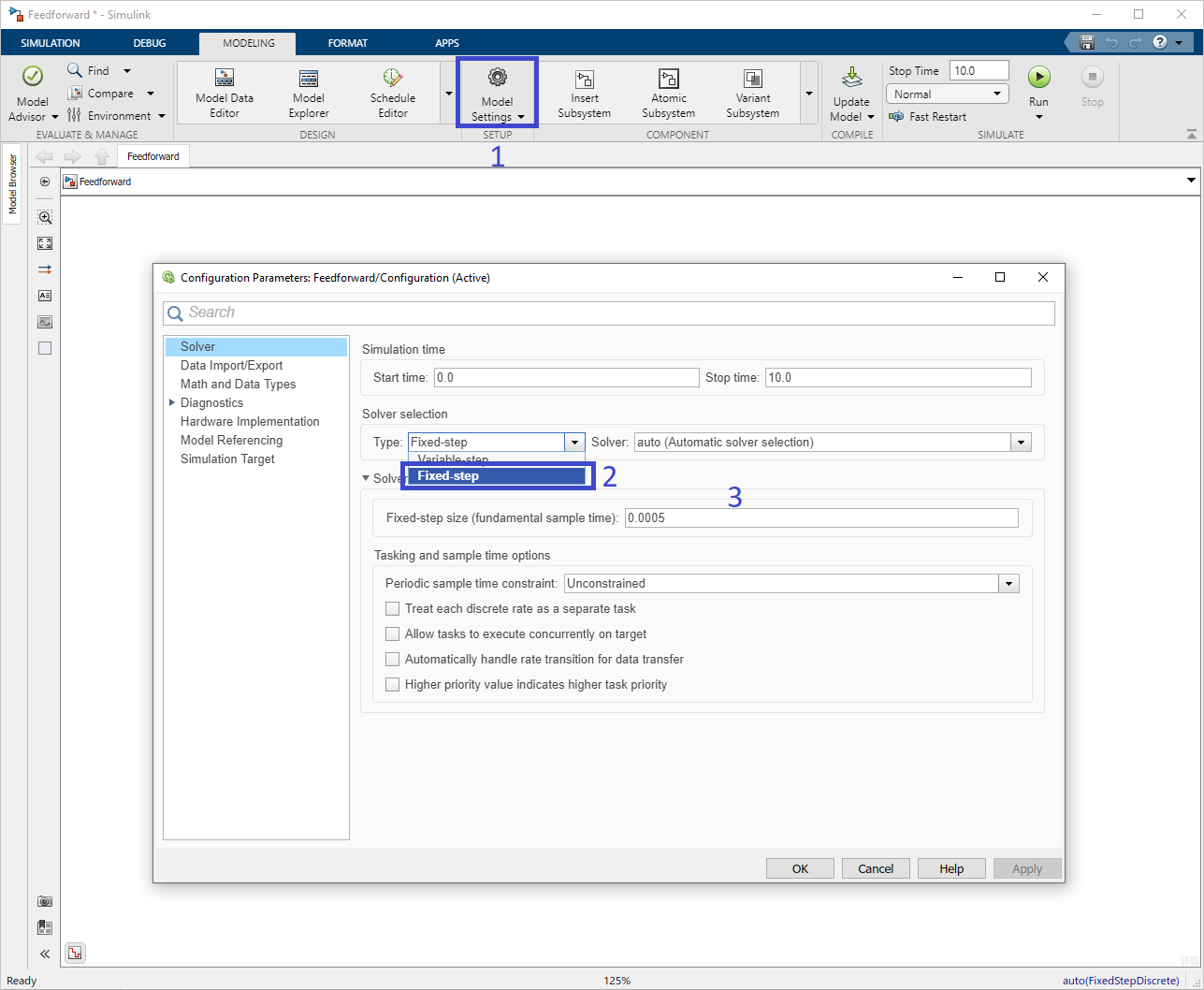
Set Simulink solver to fixed step.¶
By default, the sample time of all inputs, signals, and outputs inherit the sample time of the model.
To verify that inheritance went as expected, and that the sample time is uniform across all blocks, the sample time can be made visible via
.Note
Uniform color means uniform sampling time.
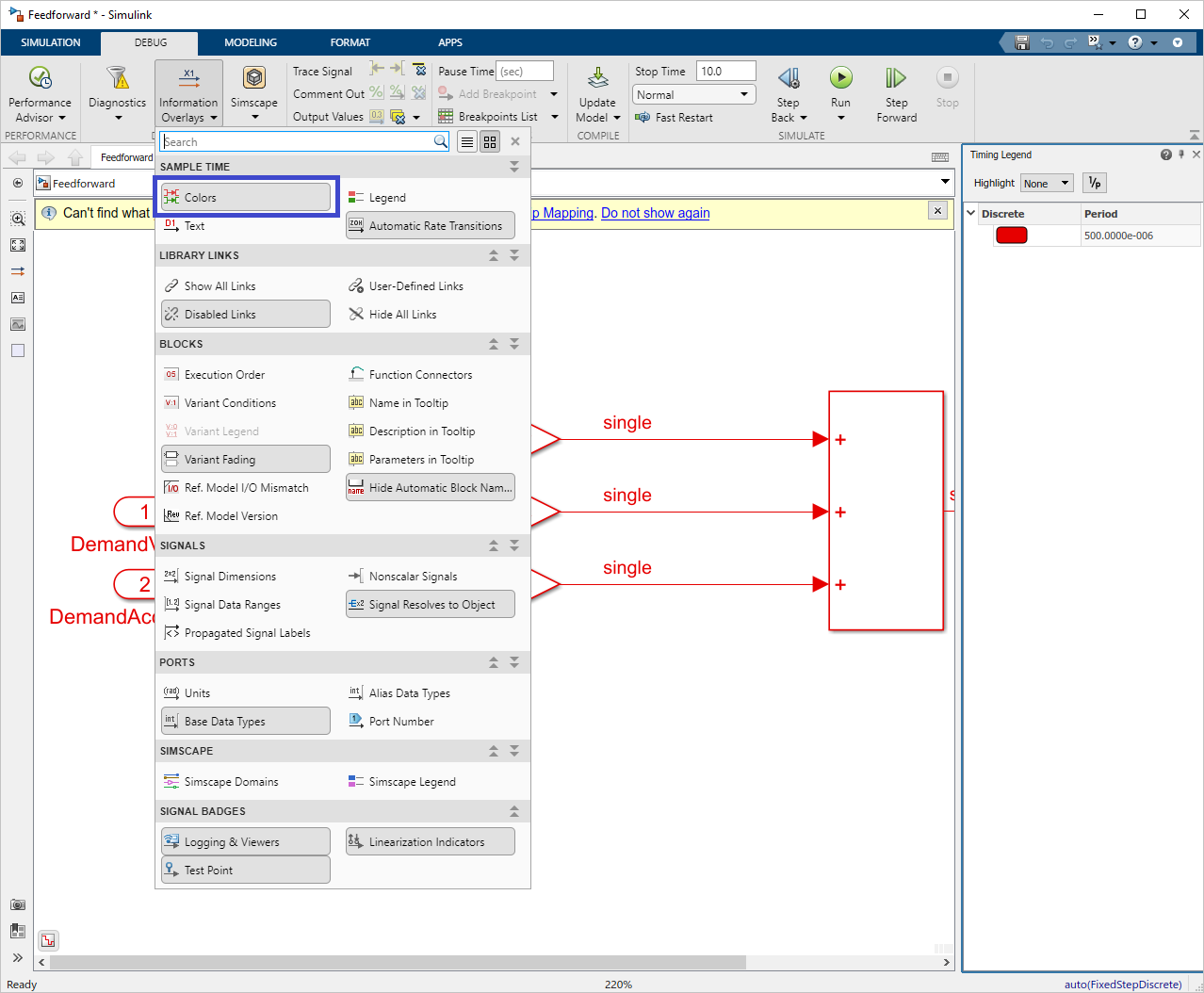
Feedforward model with visualized sample times¶
Compile model¶
Before generating a binary from the feedforward model, the model needs to be compiled first.
Save the model.
Load the following list of parameters and signals:
Kfc = PmpParameter([], [], 0.0, 'single', '', 'Coulomb friction compensation gain.'); Kfv = PmpParameter([], [], 0.0, 'single', '', 'Velocity compensation gain.'); Kfa = PmpParameter([], [], 0.0, 'single', '', 'Acceleration compensation gain.'); KfvOutput = PmpSignal([], [], '', 'Velocity compensation output.'); KfcOutput = PmpSignal([], [], '', 'Coulomb friction compensation output.'); KfaOutput = PmpSignal([], [], '', 'Acceleration compensation output.');
Note
As best practice, we recommend to put the above list in a configuration script, which is a available as
init.m
in the project files (see project download in Prerequisites).In Simulink, click on Run to compile the model.
The model is now ready to be converted into a binary file using the Code generation.
Code generation¶
The code generation generates a binary file that can be uploaded into PMP. Simulink code generation for PMP should be executed via MATLAB script.
The function PmpRunCodeGen()
allows to set the configuration parameters and generate the code for PMP from an .m
file or the command window.
The possible syntaxes for this function are:
PmpRunCodeGen(modelname,targetplatform,solver,sampleperiod)
PmpRunCodeGen(modelname,subsystemname,targetplatform,solver,sampleperiod)
PmpRunCodeGen(___,outputdir,binfilename)
Note
Code generation via the Simulink GUI is not advised nor documented, due to a large amount of required manual configurations.
Depending whether optional arguments are specified, the full model or only a specific subsystem can be built and the generated binary file can be renamed and moved to a user defined output directory.
Input |
Description |
---|---|
modelname |
String containing the name of the simulink model. |
targetplatform |
|
solver |
|
sampleperiod |
Numeric or string containing the sample period used to generate code of the model.
If the model is not dependent on the sample period, then |
subsystemname |
(optional) string containing the name of the subsystem (if unique in the model) or full model path (in case it is not unique). |
outputdir |
(optional) string containing the user defined output directory for the binary file |
binfilename |
(optional) string specifying the user defined name of the binary file. |
enumeration('Pmp.TargetPlatform')
Enumerations feature auto-completion. I.e. when typing Pmp.TargetPlatform.
and pressing the TAB key all options are displayed.
To generate code from our feedforward model, use the following command:
PmpRunCodeGen('Feedforward', PmpTargetPlatform.win_x86_64, PmpSolver.discrete, 'auto', 'CodeGenOutput', 'Feedforward-windows-x86_64.bin');
Note
The above command uses the win_x86_64
target platform to be able to load the binary on a simulated system with the PMP simulator.
The Feedforward.bin
binary file is generated in the output folder CodeGenOutput
.
The output of the building process is displayed in the Command Window. If no errors occurred the message shall be as follows:
### Successful completion of build procedure for model: Feedforward
Model version <version> generated with code generation version <releaseID>(<releaseDate>).
The version is the Simulink model version, by default, this is incremented every time the model is saved (this can be changed via the Simulink model settings if desired). For traceability, the model version can be obtained via PMP after the binary files are uploaded into the motion controller.
The binary file can now be uploaded to the (simulated) motion controller or EtherCAT SubDevice, this is described in the Deployment guide.